The most commonly used object in any project and in any programming language is String only. A string is a list of characters in order enclose by single quote, double quote or triple quotes. Python string is immutable modifications are not allow once it is create. String index starts from 0, it support both forward and backward indexing. Each character is encoded in the ASCII or Unicode character. Strings are use widely in many different applications, such as storing and manipulating text data, representing names, addresses, and other types of data that can be represent as text.
Creating a String in Python
We can create a string by enclosing the characters in single-quotes, double- quotes or triple-quotes. Triple-quotes is generally use for multiline string.
Example of Creating a sting in Python#single quotes key1 = 'Basic Engineer' print('single quotes') print(key1) #double quotes key2 = "Basic Engineer" print("double quote") print(key2) #triple quotes key3 = '''Basic Engineer Basic Engineer Basic Engineer Basic Engineer Basic Engineer Basic Engineer Basic Engineer''' print('''triple quotes''') print(key3)
Let’s run the code
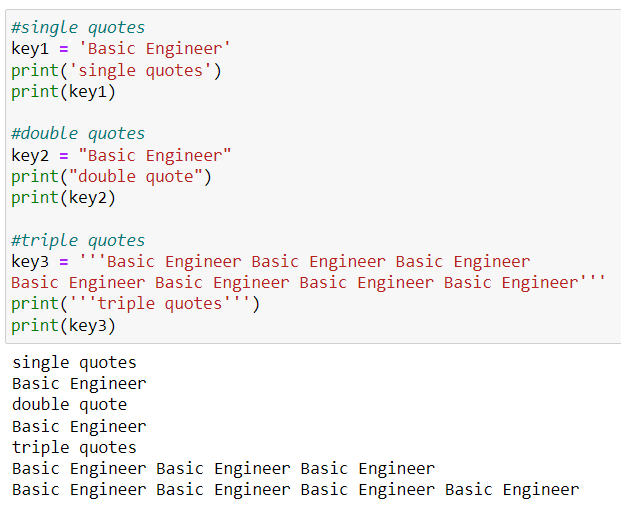
Strings Splitting and indexing in Python
The slice operator [] is use to access the individual characters of the string and colon operator use to access the substring from the given string. The indexing of the Python strings starts from 0.
A Example of Strings Splitting and indexing in Python
Example of Strings Splitting and indexing in Python#index str = "BASIC" print(str[0]) print(str[1]) print(str[2]) print(str[3]) print(str[4]) #sliceing str = "BASICENGINEER" print(str[0:]) print(str[3:7]) print(str[6:10]) #sliceing str = "BASICENGINEER" print(str[-1:]) print(str[-3:-7]) print(str[-6:-10])
Let’s run the code
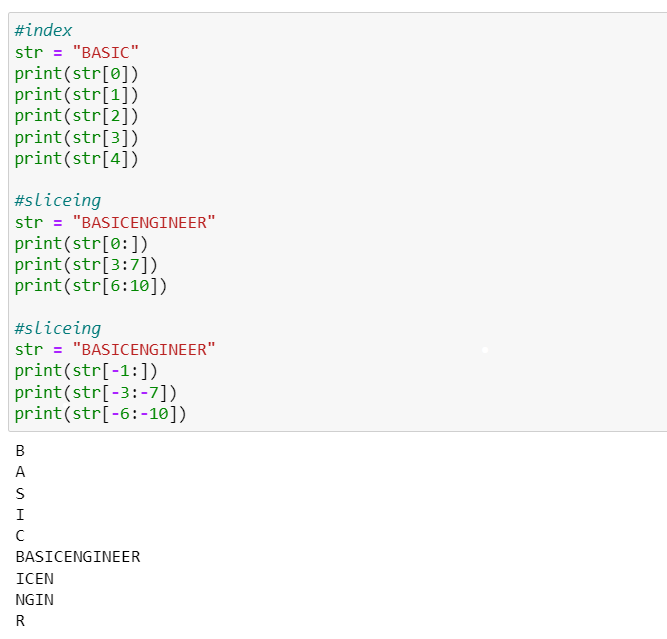
Escape Sequence
Escape Sequence | Description |
\newline | It ignores the new line. |
\\ | Backslash |
\’ | Single Quotes |
\\” | Double Quotes |
\a | ASCII Bell |
\b | ASCII Backspace |
\f | ASCII Formfeed |
\n | ASCII Linefeed |
\r | ASCII Carriege Return |
\t | ASCII Horizontal Tab |
\v | ASCII Vertical Tab |
Example of Escape Sequence
A Example of Escape Sequenceprint("basic engineer \basic engineer2 \basic engineer3") print("\\basic engineer") print('\'basic engineer') print("\"basic engineer") print("\a basic engineer") print("basic \b engineer") print("basic \f engineer") print("basic \n engineer") print("basic \r engineer") print("basic \t engineer") print("basic \v engineer")
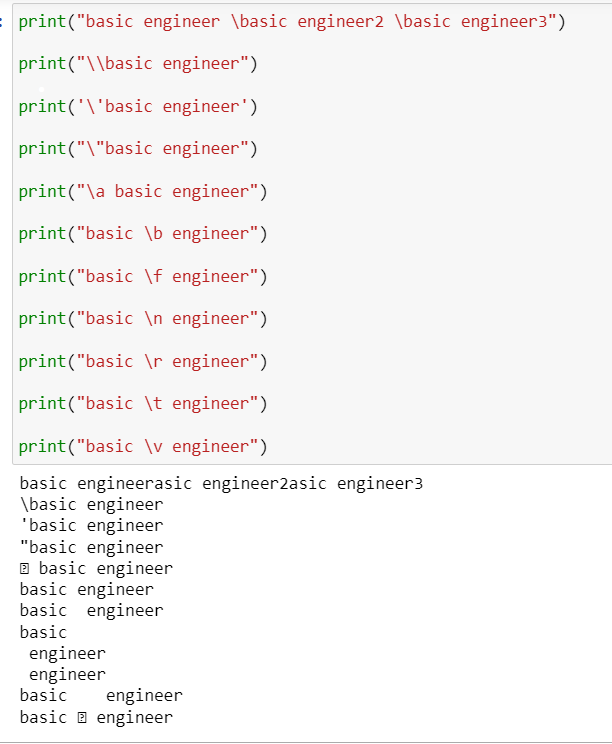
Method of String
Methods | Description |
---|---|
upper() | converts the string to uppercase |
lower() | converts the string to lowercase |
partition() | returns a tuple |
replace() | replaces substring inside |
find() | returns the index of first occurrence of substring |
rstrip() | removes trailing characters |
split() | splits string from left |
startswith() | checks if string starts with the specified string |
isnumeric() | checks numeric characters |
index() | returns index of substring |
swapcase() | Inverts case for all letters in string. |
min() | returns the min alphabetical character from the string. |
max() | returns the max alphabetical character from the string. |
Example of Method of String in Python
A Example of Method of String in Python#UPPERCASE message = 'basic engineer' print(message.upper()) #lowercase message = 'BASIC ENGINEER' print(message.lower()) # replace text = 'basic engineer' replaced_text = text.replace('basic', 'hello') print(replaced_text) # split key = 'hello-student-basic-engineer' print(key.split('-'))
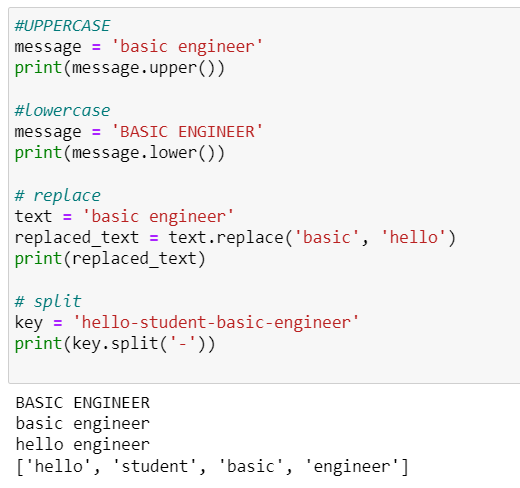
If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about Python Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments