In this tutorial, we will learn about the Module in Python. Its types, and how to use them with the help of examples
Module is the collection of function we define function within module because of we want to use the certain function in any python program simple by importing the module & use the related function.
Steps for Creating Module in Python
- Create a new program File.
- Define the function one by one in program file.
- Save the file as – ModuleName.py
Example of Module in Python
Code# mymodule.py def my_function(): print("This is a function in mymodule") class MyClass: def __init__(self): print("This is a class in mymodule") my_variable = "This is a variable in mymodule"
Let’s run the code
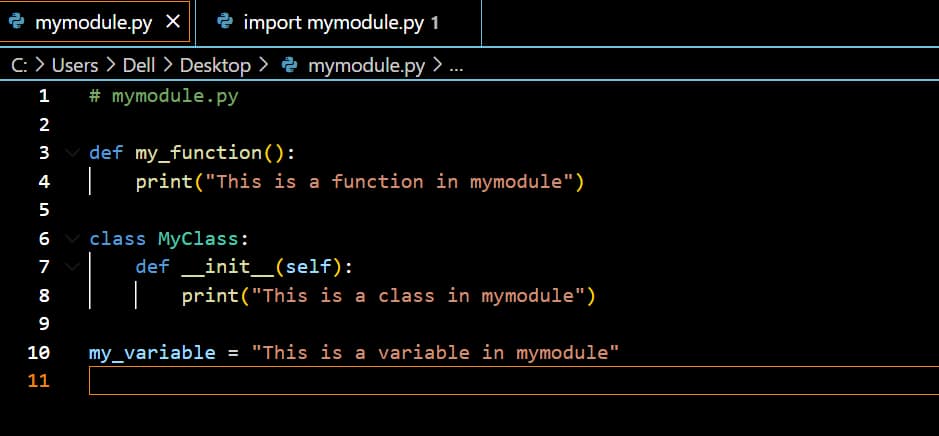
codeimport mymodule mymodule.my_function() # Output: This is a function in mymodule obj = mymodule.MyClass() # Output: This is a class in mymodule print(mymodule.my_variable) # Output: This is a variable in mymodule
Let’s run the code
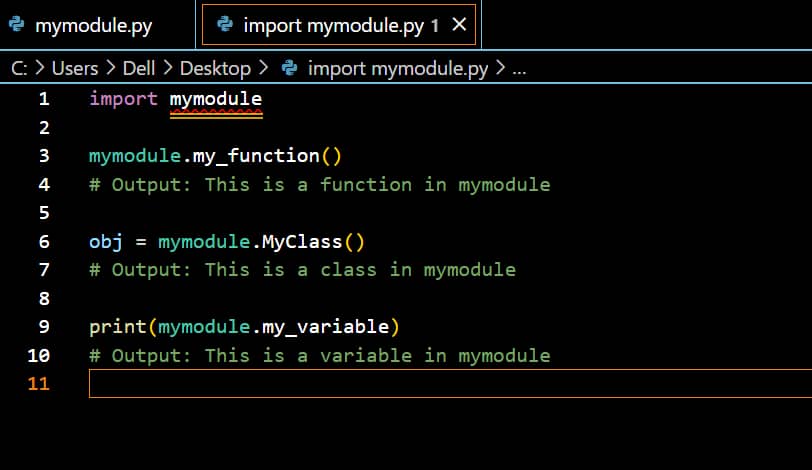
Output Value

Constructor
Constructor is the special member function provided by OOPs concept. It is special because of invoke or call automatically when you crate an object of the class. Construction can be call only one time for each object of the class & hence. It can be use for variable initialization, resource opening like file Opening/ database opening, etc. The task of constructors is to initialize and assign value to the data members of the class, when an object of the class is created.
Syntax of Constructor in Python
Syntax of Constructor in Pythonclass ClassName: def __init__(self,variables...): ##body
In Python, __init__() is the Constructor non-parameterized but cannot return value. It is always be public.
Example of Constructor in python
A Example of Constructor in pythonclass Addition: def __init__(self, a, b): self.key1 = a self.key2 = b def calculate(self): print(self.key1 + self.key2) obj = Addition(5632, 58745) obj.calculate()
Let’s run the code

Destructor In Python
Python Destructor is also a special method that gets executed automatically when an object exit from the scope. To destroy the object use del. Destructors are called when an object gets destroyed, it is opposite to constructors. When we destroy the object the del() function executed. When multiple reference variables are pointing to same object , if all reference variable count will become zero then only del() will be executed.
Syntax of Destructor In Python
Syntax of Destructor In Pythonclass ClassName: def __del__(self,): ##body
Example
Example of Destructor In Pythonclass MyClass: def __init__(self): print("Object created") def __del__(self): print("Object deleted") obj = MyClass() del obj
Let’s run the code

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about Python Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments