In this tutorial, we will learn about the Function in Python. Its types, and how to use them with the help of examples.
Function is a Sub-program within program that perform specific operation within program. After performing an operation function returns a value which may the result of operation.
There are two keywords
- def
- It is a mandatory
- return
- It is a optional
Advantages of functions
- User-defined functions are reusable code blocks; they only need to be written once, then they can be used multiple times.
- These functions are very useful, from writing common utilities to specific business logic.
- The code is usually well organized, easy to maintain, and developer-friendly.
- A function can have dif types of arguments & return value.
How to Create the function in Python?
- Declare the function with the keyword def followed by the function name.
- Write the arguments inside the opening and closing parentheses of the function, and end the declaration with a colon.
- Add the program statements to be execute.
- End the function with/without return statement.
Syntax
def functionname( parameters ): "function_docstring" function_suite return [expression]
Types
There are two types of functions
- Library Functions
- User define function (UDF)
Library Functions
There function provided by the programming language for performing specific operation within program. Library functions comes under the concept of module.
User define function
This function is develop by user itself within program/module for performing specific task. In Python, a user-defined function is created using the def
keyword, followed by the function name and parentheses that may include parameters. The code block within the function is indented and begins with a colon.
Example of User define functiondef greet(name): print("Hello, " + name ) greet("Basic Engineer")
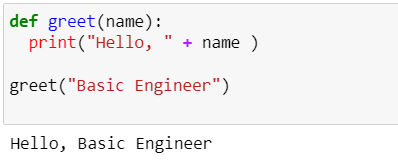
Calling Function
A Function runs only when we call it, function can not run on its own. Defining a function only gives it a name, specifies the parameters that are to be include in the function and structures the blocks of code.
A Example of Calling Functiondef key(): print("Basic Engineering") key()
Let’s run the code
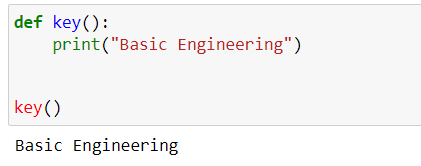
Pass by Reference vs. Pass by Value
All parameter in the python programming language are passes by reference. It means if you change what a parameter refer to within a function, the change also refers back in the calling function.
The Example of Pass by Reference and Pass by Value
Example of Pass by Reference and Pass by Valuedef square( key ): squares = [ ] for l in key: squares.append( l**2 ) return squares bon= [5,12, 28]; result = square(bon) print( "Squares of the list are: ", result )
Let’s run the code
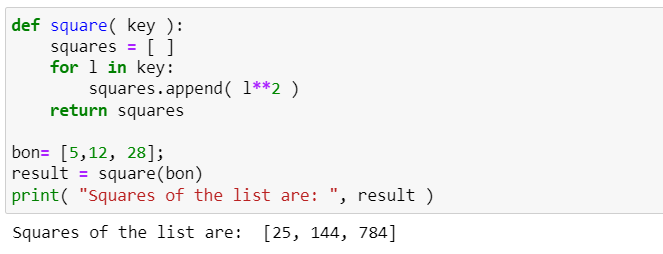
If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about Python Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments