In this tutorial, we will learn about the Python Loop Control Statements. Its types, and how to use them with the help of examples.
Python Loop control statements change execution from their normal sequence. When execution leaves a scope, all automatic objects that were create in that scope are destroy.
There are three types of Loops Control statement
- Continue Statement
- Break Statement
- Pass Statement
Continue Statement
Continue statement is use in a loop to go back to the beginning of loop. This statement can be used in both while and for loops.
Syntax
Syntax Continue Statementcontinue
Flow Diagram of Continue Statement in Python
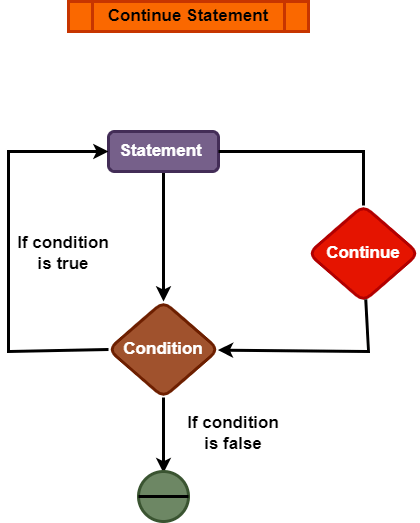
Example of Python continue Statement with for Loop
Continue Statement with for Loop#continue for loop for i in range(10): if i == 5: continue print(i)
Let’s run the code
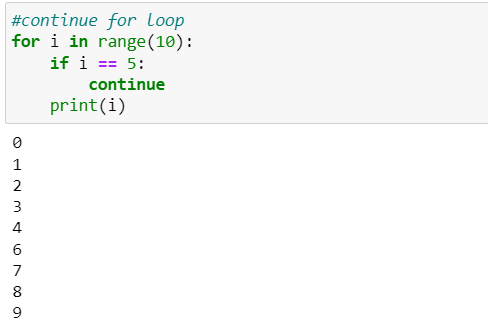
Example Python continue Statement with while Loop
Continue Statement with while Loop#continue while loop num = 3 while num < 15: num += 1 if (num % 2) ==0: continue print(num)
Let’s run the code
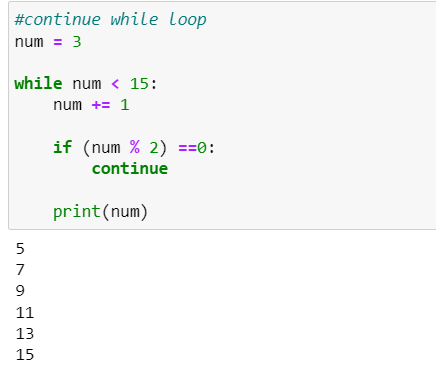
Break Statement
The most common use for break is when some external condition is triggered requiring a hasty exit from a loop. Break statement is use to jump out of loop to process the next statement in the program. This statement can be use in both while and for loops.
Syntax
Syntax of Break Statement in Pythonbreak
Flow Diagram of Break Statement in Python

The Example of Python break Statement with for Loop
Python break Statement with for Loopfor i in range(10): if i == 5: break print(i)
Let’s run the code

The Example of Python break Statement with while Loop
Example of Python break Statement with while Loopi = 1 while i <= 20: print('9 * ',(i), '=',9 * i) if i >= 10: break i = i + 1
Let’s run the code

Pass Statement
The statement is a null statement.
Syntax
Syntax of Pass Statementpass
Example of Pass Statement in Python
Example of Pass Statement in Pythonfor char in 'basicengineer': if (char == 'e'): pass print("current character: ",char)
Let’s run the code

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about Python Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments