It is a variable which store the address of another variable is knows as C Pointer. Variable can be of any data type like int, char, function, array, or any other Pointer. The size of the pointer depends on the architecture.
Syntax
datatype *var_name
Sample Example of Pointer
#include <stdio.h> int main () { int rom; char com[56]; printf("Address of var1 variable: %x\n", &rom ); printf("Address of var2 variable: %x\n", &com ); return 0; }
Output
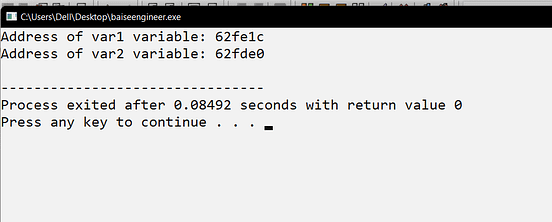
How to work pointer in c
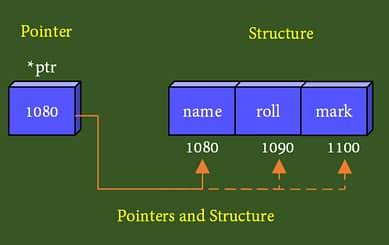
Advantage of Pointer
- Pointer reduces the code and improves the performance.
- Return multiple values from a function.
- Access any memory location.
Usage of pointer
- Dynamic memory allocation
- Dynamically allocate memory using malloc() and calloc() functions
- Arrays, Functions, and Structures
- It wide use in arrays, functions, and structures.
NULL Pointer
A pointer that is not assign any value but NULL is known as the NULL pointer. The NULL pointer is a constant with a value of zero define.
Example of Null Pointer
#include <stdio.h> int main () { int *ptr = NULL; printf("The value of ptr is : %x\n", ptr ); return 0; }
Output

Pointer Arithmetic in C
A pointer in c is an address, which is a numeric value. It is perform arithmetic operations on the pointers like addition, subtraction, Increment, etc.
Types of Pointer Arithmetic in C
- Increment
- Decrement
- Addition
- Subtraction
- Comparison
Incrementing Pointer in C
The Rule to increment
new_address= current_address + i * size_of(data type)
- 32-bit
- 32-bit int variable, it will be increment by 2 bytes.
- 64-bit
- 64-bit int variable, it will be increment by 4 bytes.
Example
#include <stdio.h> const int MAX = 5; int main () { int rom[] = {1000, 2000, 3000, 4000, 5000}; int i, *ptr; ptr = rom; for ( i = 0; i < MAX; i++) { printf("Address of rom[%d] = %x\n", i, ptr ); printf("Value of rom[%d] = %d\n", i, *ptr ); ptr++; } return 0; }
Output

Decrementing Pointer in C
new_address= current_address – i * size_of(data type)
- 32 bit
- 32-bit int variable, it will be decremented by 2 bytes
- 64 bit
- 64-bit int variable, it will be decremented by 4 bytes.
Example
#include <stdio.h> const int MAX = 5; int main () { int var[] = {100, 200, 300, 400, 500}; int i, *ptr; ptr = &var[MAX-1]; for ( i = MAX; i > 0; i--) { printf("Address of var[%d] = %x\n", i-1, ptr ); printf("Value of var[%d] = %d\n", i-1, *ptr ); ptr--; } return 0; }
Output

C Pointer Addition
Syntax
new_address= current_address + (number * size_of(data type))
32-bit
For 32-bit int variable, it will add 2 * number.
64-bit
For 64-bit int variable, it will add 4 * number.
Example
Input
#include<stdio.h> int main(){ int number=25; int *p; p=&number; printf("Address of p variable is %u \n",p); p=p+3; printf("After adding 3: Address of p variable is %u \n",p); return 0; }
Output

C Pointer Subtraction
new_address= current_address – (number * size_of(data type))
32-bit
For 32-bit int variable, it will subtract 2 * number.
64-bit
For 64-bit int variable, it will subtract 4 * number.
Example
Input Value
#include<stdio.h> int main(){ int number=25; int *p; p=&number; printf("Address of p variable is %u \n",p); p=p-3; printf("After subtracting 3: Address of p variable is %u \n",p); return 0; }
Output

Array of pointers
1.Example
Input values
#include <stdio.h> const int MAX = 4; int main () { int var[] = {15, 18, 20, 22}; int i; for (i = 0; i < MAX; i++) { printf("Value of var[%d] = %d\n", i, var[i] ); } return 0; }
Output

2.Example
Input value
#include <stdio.h> const int MAX = 4; int main () { int var[] = {10, 15, 20, 25 }; int i, *ptr[MAX]; for ( i = 0; i < MAX; i++) { ptr[i] = &var[i]; /* assign the address of integer. */ } for ( i = 0; i < MAX; i++) { printf("Value of var[%d] = %d\n", i, *ptr[i] ); } return 0; }
Output

3.Example
Input value
#include <stdio.h> const int MAX =4 ; int main () { char *names[] = { "raj", "rohit", "rohni", "sohil" }; int i = 0; for ( i = 0; i < MAX; i++) { printf("Value of names[%d] = %s\n", i, names[i] ); } return 0; }
Output

Pointer to Pointer (Double Pointer)
A pointer to a pointer is a form of multiple indirection, or a chain of pointers. Normally, a pointer contains the address of a variable is knows as Pointer to Pointer. The first pointer contains the address of the second pointer, which points to the location that contains the actual value.

Syntax
int **var;
Example
Input value
#include <stdio.h> int main () { int var; int *ptr; int **pptr; var = 1254; ptr = &var; pptr = &ptr; printf("Value of var = %d\n", var ); printf("Value available at *ptr = %d\n", *ptr ); printf("Value available at **pptr = %d\n", **pptr); return 0; }
Output

Passing pointers to functions
The Memory location of the variable is passes to the parameters in the function, and then the operations are perform. The functions definitions accepts these addresses using pointer, addresses are store using pointers.
1. Example
Input value
#include <stdio.h> #include <time.h> void getminutes(unsigned long *par); int main () { unsigned long min; getminutes( &min ); printf("Number of minute: %ld\n", min ); return 0; } void getminutes(unsigned long *par) { *par = time( NULL ); return; }
Output

2.Example
Input value
#include <stdio.h> double getAverage(int *arr, int size); int main () { int balance[7] = {10, 20, 30, 40, 50, 60, 70}; double avg; avg = getAverage( balance, 7 ) ; printf("Average : %f\n", avg ); return 0; } double getAverage(int *arr, int size) { int i, sum = 0; double avg; for (i = 0; i < size; ++i) { sum += arr[i]; } avg = (double)sum / size; return avg; }
Output

Return pointer from functions
Return a pointer to the local variable, static variable, and dynamically allocated memory as well as.
Syntax
int * myFunction() { . . . }
Example
Input value
#include <stdio.h> #include <time.h> int * getRandom( ) { static int r[15]; int i; srand( (unsigned)time( NULL ) ); for ( i = 0; i < 7; ++i) { r[i] = rand(); printf("%d\n", r[i] ); } return r; } int main () { int *p; int i; p = getRandom(); for ( i = 0; i < 7; i++ ) { printf("*(p + [%d]) : %d\n", i, *(p + i) ); } return 0; }
Output

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about C Programming language please Wikipedia click here .
Stay Connected Stay Safe, Thank you
0 Comments