C language Tutorial with programming approach for beginners and professionals, helps you to understand the C language tutorial easily. Our C tutorial explains each or every topic with example.
The C programing language is a procedural and general- purpose language that provides low-level access to System memory is knows as C programming language.
Control Statement
C programming language provides the following types of Control statements.
- If statement
- If-else statement
- If else-if ladder
- Nested if
If statement
It is use to specify a block of code to be execute, if specified condition is true is knows as If Statement.
Flow Diagram
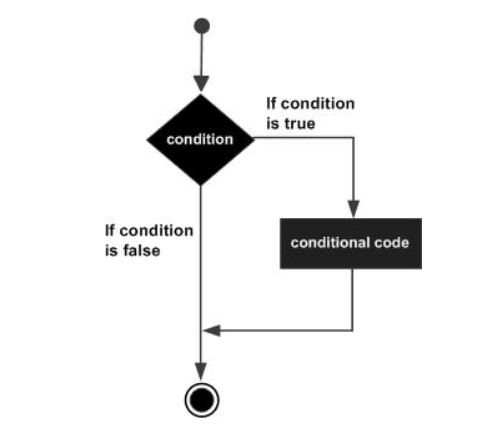
Syntax
- if (condition) {
- // block of code to be execute if the condition is true
- }
Example
#include <stdio.h> int main() { int x, y, z; printf("Enter three numbers?"); scanf("%d %d %d",&x,&y,&z); if(x>y && x>z) { printf("%d is largest",x); } if(y>x && y > z) { printf("%d is largest",y); } if(z>x && z>y) { printf("%d is largest",z); } if(x == y && x == z) { printf("All are equal"); } }
Output
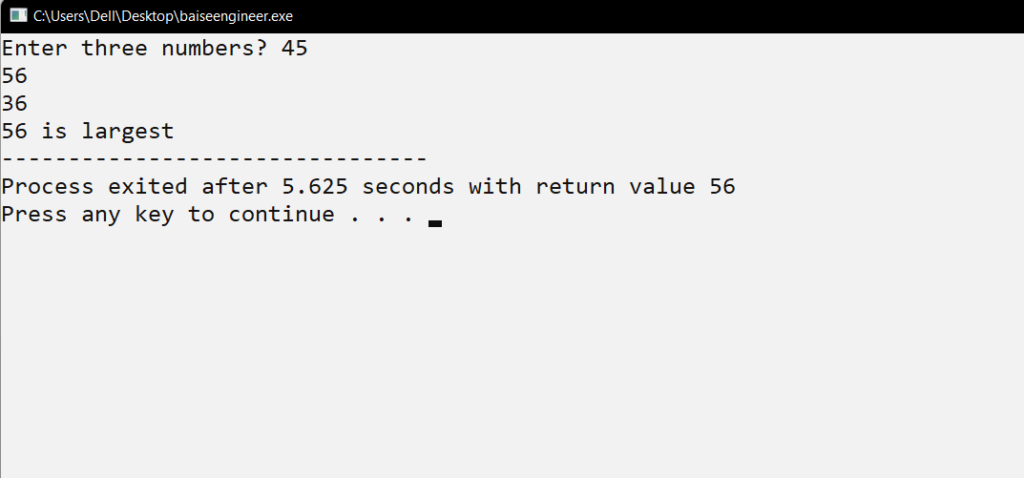
if…else statement
It is use to specify a new condition to test, if first condition id false is knows as if…else statement.
Flow diagram of if…else statement
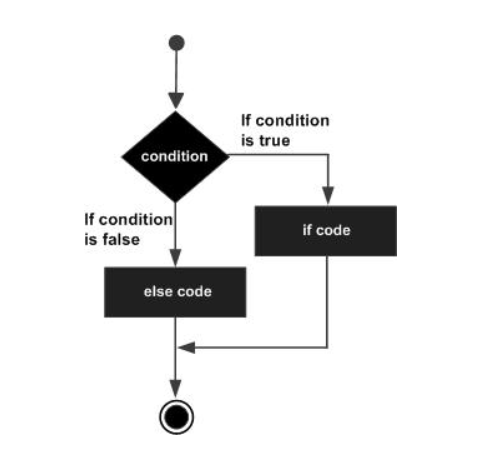
Syntax
- if(expression){
- //code to be execute if condition is true
- }else{
- //code to be execute if condition is false
- }
Example
#include<stdio.h> int main(){ int num=0; printf("enter a number:"); scanf("%d",&num); if(num%2==0){ printf("%d is even number",num); } else{ printf("%d is odd number",num); } return 0; }
Output
Enter the even number

Enter the odd number
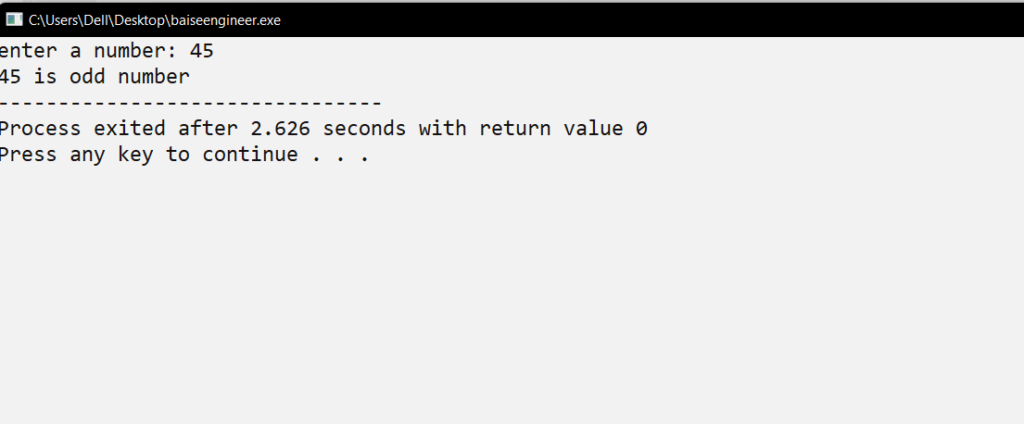
If else-if ladder Statement
if-else-if ladder statement, if a condition is true then the statements define in the if block will be execute, otherwise if some other condition is true then the statements define in the else-if block will be execute, at the last if none of the condition is true then the statements define in the else block will be execute is knows as If else-if ladder Statement.
Flow Diagram If else-if ladder Statement
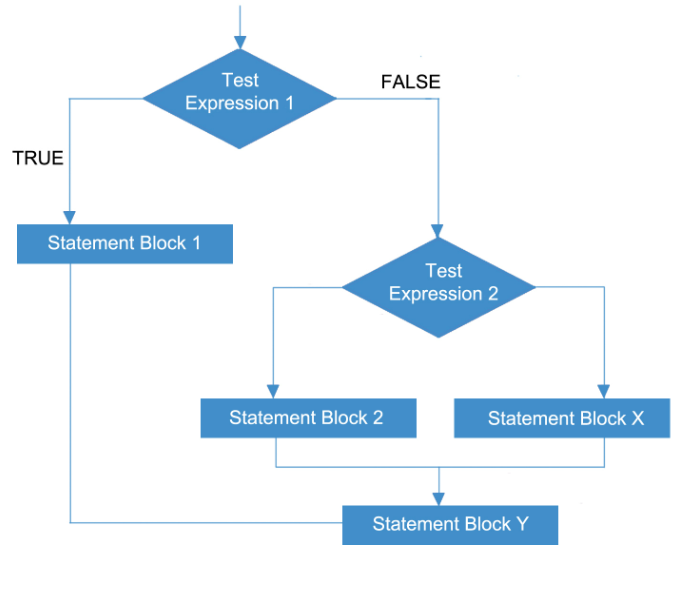
Syntax
if(condition1){
//code to be executed if condition1 is true
}else if(condition2){
//code to be executed if condition2 is true
}
else if(condition3){
//code to be executed if condition3 is true
}
...
else{
//code to be executed if all the conditions are false
}
Example
#include<stdio.h> int main(){ int num=0; printf("enter a number:"); scanf("%d",&num); if(num==15){ printf("number is equals to 15"); } else if(num==75){ printf("number is equal to 75"); } else if(num==100){ printf("number is equal to 150"); } else{ printf("number is not equal to 15, 75 or 150"); } return 0; }
Output
Number is not equal to 15, 75, or 150
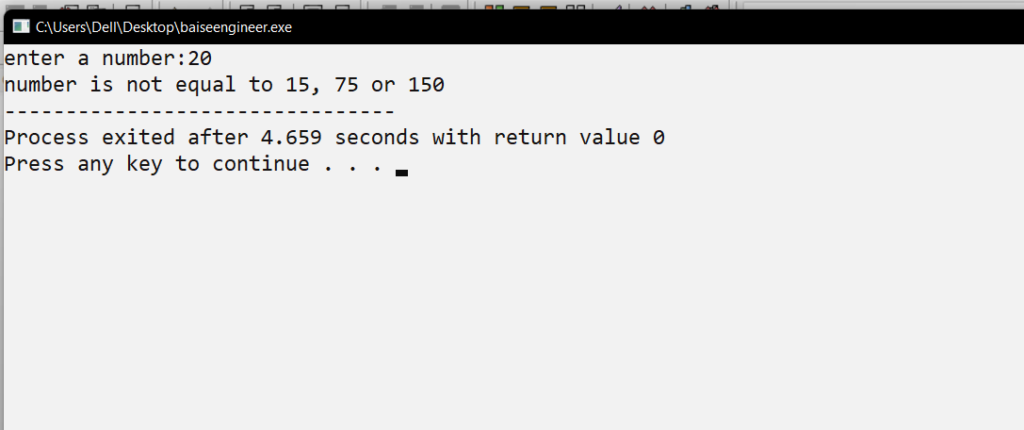
Number is equal to 15, 75, or 150

Nested if statements
It means that if statement inside another if statement is knows as Nested if statements
Syntax
if( boolean_expression 1) {
/* Executes when the boolean expression 1 is true */
if(boolean_expression 2) {
/* Executes when the boolean expression 2 is true */
}
}
Example
#include <stdio.h> int main () { int x = 520; int y = 720; if( x == 520 ) { if( y == 720 ) { printf("Value of x is 520 and y is 720\n" ); } } printf("Exact value of x is : %d\n", x ); printf("Exact value of y is : %d\n", y ); return 0; }
Output

Switch statement
This statements in the multiple cases for the different values of a single variable is knows as Switch Statement.
Flow Diagram
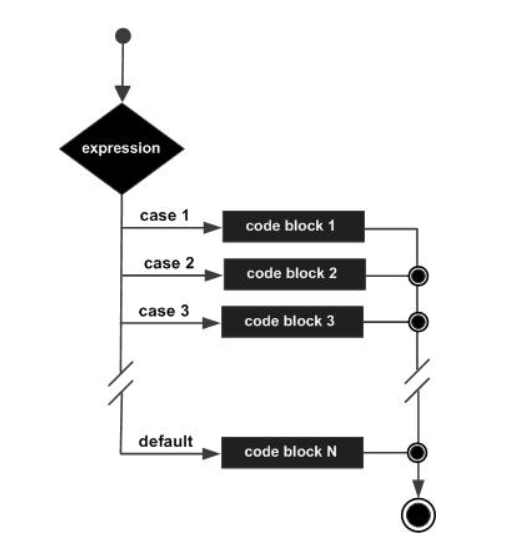
Syntax
switch(expression) {
case constant-expression :
statement(s);
break; /* optional */
case constant-expression :
statement(s);
break; /* optional */
/* you can have any number of case statements */
default : /* Optional */
statement(s);
}
Example
#include<stdio.h> int main(){ int num=0; printf("enter a number:"); scanf("%d",&num); switch(num){ case 15: printf("number is equals to 15"); break; case 75: printf("number is equal to 75"); break; case 150: printf("number is equal to 150"); break; default: printf("number is not equal to 15, 75 or 150"); } return 0; }
Output
Number is not equal to 15, 75, or 150
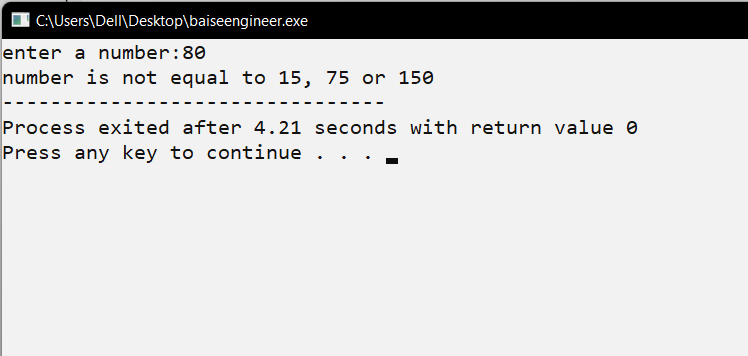
Number is equal to 15, 75, or 150

Loop
A loop is use to repeat a block of code until the specifies condition is met is called Loop.
Types of C Loops
There are three types of Loop
- do while loop
- while loop
- for loop
Do while Loop
It creates a structure loop that execute as long as a specifies condition is true at the
end of each pass through the loop is knows as Do While loop.
Flow Diagram of Do while Loop

Syntax
do { statement(s); } while( condition );
Example
#include <stdio.h> int main () { int x = 1; do { printf("value of x: %d\n", x); x = x + 1; }while( x < 10 ); return 0; }
Output
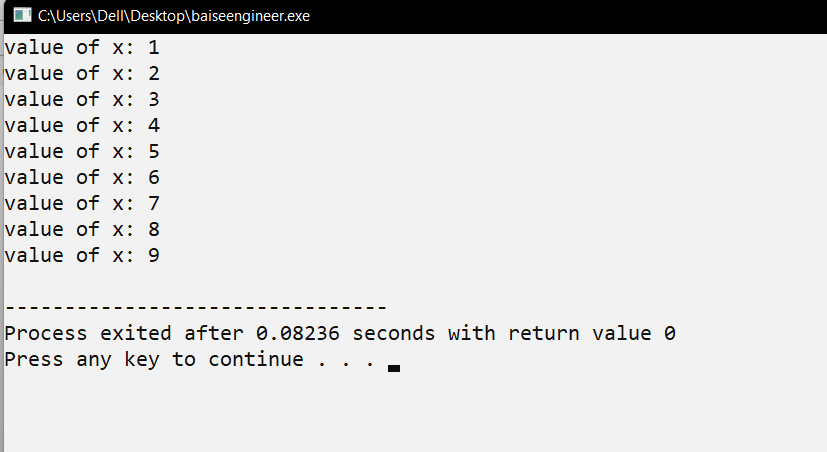
While loop
It allow to repeatedly run the same block of code until a condition is met . While
loop has one control condition, an execute as long the condition is true is knows as while Loop.
Flow diagram

Syntax
while(condition) { statement(s); }
Example
#include <stdio.h> int main () { int x = 0; while( x < 10 ) { printf("value of x: %d\n", x); x++; } return 0; }
Output
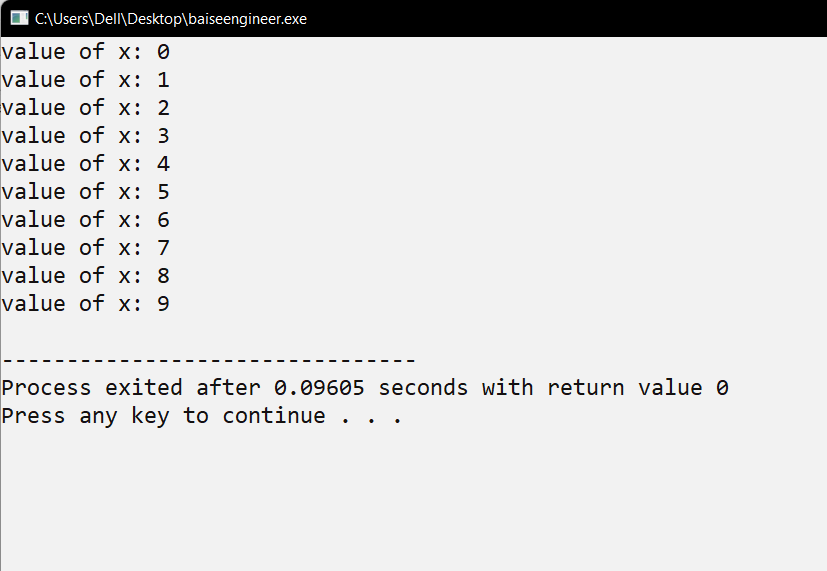
For loop
It is a repetition control structure that allows you to efficiently write a loop that needs to
execute a specific number of times is knows as For loop.
Flow Diagram
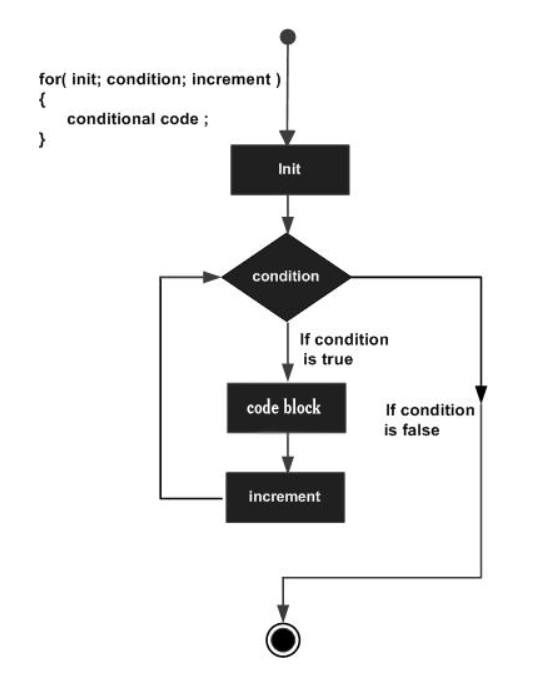
Syntax
for ( init; condition; increment ) { statement(s); }
Example
#include <stdio.h> int main () { int x; for( x = 1; x < 20; x = x + 2 ){ printf("value of x: %d\n", x); } return 0; }
Output

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about C Programming language please Wikipedia click here .
Stay Connected Stay Safe, Thank you
0 Comments