Vector is the pre-defined class provided by java for making heterogeneous list. Java introduce vector in ver5 and later. It is define under java.util package. Vector is like the dynamic array.
Methods of vector
addElement() Method
This adds a new value of any type in vector list.
Syntax
obj.addElement(value);
Example of addElement() Method in Java
Input Value
addElement() Method in Javaimport java.util.Vector; public class vectorg { public static void main(String arg[]) { Vector<Integer> a = new Vector<>(3); a.add(25); a.add(-35); a.add(45); System.out.println("Vector: "+a); a.addElement(-65); System.out.println("after vector: "+a); } }
Let’s Run the code
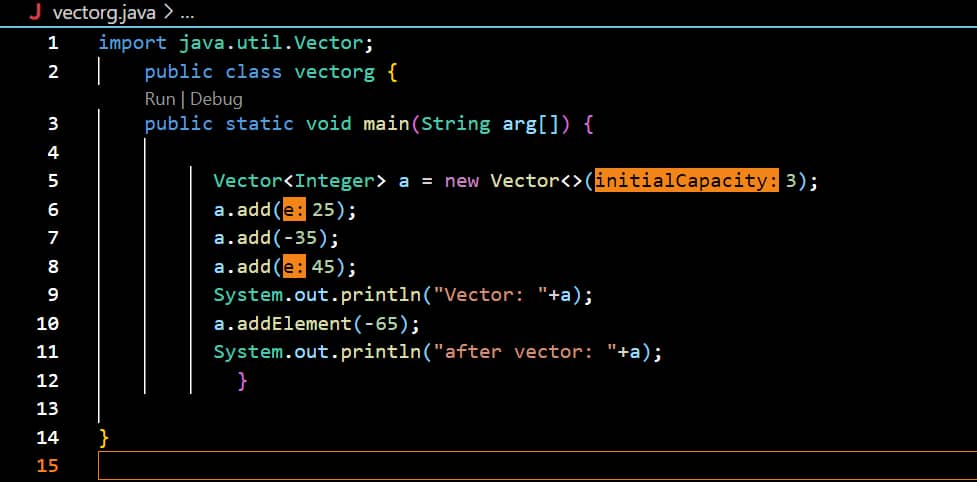
Output Value

size() Method
This returns the size of the vector means how many elements in vector list
Syntax
obj.size();
Example of size() Method in Java
Input Value
size() Method in Javaimport java.util.*; public class vectorg { public static void main(String args[]) { Vector<String> a = new Vector<String>(); a.add("hello"); a.add("basic"); a.add("student"); a.add("engineer"); System.out.println("Vector: " + a); System.out.println("The size is: " + a.size()); } }
Let’s Run the code

Output Value

elementAt() Method
This return the given index position value of the vector list.
Syntax
obj.element(index);
Example of elementAt() Method in Java
Input Value
elementAt() Method in Javaimport java.util.*; public class vectorg { public static void main(String args[]) { Vector < String > colors = new Vector < String > (); colors.add("Red"); colors.add("Blue"); colors.add("Yellow"); colors.add("gray"); System.out.println("Element position = " +colors.elementAt(1)); System.out.println("Element position = " +colors.elementAt(3)); } }
Let’s Run the code

Output Value

insertElementAt() Method
This methods inserts the new item at specified position in vector list
Syntax
insertElementAt(item,pos);
Example of insertElementAt() Method in Java
Input Value
insertElementAt() Method in Javaimport java.util.*; public class vectorg { public static void main(String args[]) { Vector<Integer> a = new Vector<>(); a.add(5); a.add(10); a.add(15); a.add(20); a.add(25); System.out.println("before = "+a); a.insertElementAt(40, 4); System.out.println("after= "+a); } }
Let’s Run the code

Output Value

removeElement() Method
This removes the given item element from Vector list.
Syntax
obj.removeElement(item);
Example of removeElement() Method in Java
Input Value
removeElement() Method in Javaimport java.util.*; public class vectorg { public static void main(String args[]) { Vector < Integer > a = new Vector < > (); a.add(5); a.add(10); a.add(15); a.add(20); a.add(25); System.out.println("total Values: " +a); System.out.println("Remove: "+a.remove((Integer)15)); System.out.println("vector: " +a); } }
Let’s Run the code

Output Value

removeElementAt() Method
This removes the given position item from vector list.
Syntax
obj.removeElementAt(pos);
Example of removeElementAt() Method in Java
Input Value
removeElementAt() Method in Javaimport java.util.*; public class vectorg { public static void main(String args[]) { Vector<String> a = new Vector<String>(); a.add("5"); a.add("10"); a.add("15"); a.add("20"); a.add("25"); System.out.println("Vector: " + a); System.out.println("size : " + a.size()); a.removeElementAt(4); System.out.println("Final: " + a); System.out.println("size: " + a.size()); } }
Let’s Run the code

Output Value

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about JAVA Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments