This package (“Tk interface”) is the standard Python interface. There are available on most Unix platforms, including macOS, as well as on Windows systems. Tkinter is not a thin wrapper. Python with tkinter is the fast and easy way to create the GUI (Graphical User Interface) applications. Tkinter for creating graphical user interface for desktop base applications.
Tkinter top-level window can be create by using the following steps :-
- import the Tkinter module.
- Create the main application window.
- Add the widgets such like labels, buttons, frames, etc. to the window.
- Call the main event loop so that the actions can take place on the user’s computer screen.
Example
from tkinter import *
top = Tk()
top.mainloop()
Output
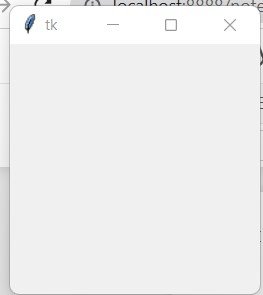
Tkinter Widgets
There are various widgets
- Button
- Canvas
- Checkbutton
- Entry
- Frame
- Label
- ListBox
- Menubutton
- Menu
- Message
- Radiobutton
- Scale
- Scrollbar
- Text
- TopLevel
- LabelFrame
- Messagebox
Button Widgets
The Button is use to add various kinds of buttons to the python application is knows as Button Widgets.
Syntax
Button (master, option = value)
import tkinter as tk
r = tk.Tk()
button = tk.Button(r, text=’Plz click me’, width=25, command=r.destroy)
button.pack()
r.mainloop()
Output

CheckButton
The Checkbutton is use to display the CheckButton on the window is knows as CheckButton.
Syntax
CheckButton (master, option = value)
Example
from tkinter import *
root = Tk()
v1 = IntVar()
Checkbutton(root, text=’HTML’,width =35, variable=v1).grid(row=0, sticky=S)
v2 = IntVar()
Checkbutton(root, text=’CSS’,width =35, variable=v2).grid(row=1, sticky=S)
v3 = IntVar()
Checkbutton(root, text=’JAVA’,width =35, variable=v3).grid(row=2, sticky=S)
mainloop()
Output
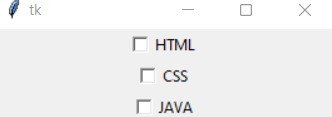
RadioButton
The user is provided with various options and the user can select only one option among them is know as RadioButton
Syntax
RadioButton (master, option = values)
Example
from tkinter import *
root = Tk()
v = IntVar()
Radiobutton(root, text=’math’,width =35, variable=v, value=1).pack(anchor=W)
Radiobutton(root, text=’bio’,width =35, variable=v, value=2).pack(anchor=W)
Radiobutton(root, text=’others’,width =35, variable=v, value=3).pack(anchor=W)
mainloop()
Output
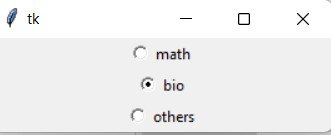
Python Tkinter Geometry
Tkinter geometry specifies the method by using which, the widgets are represented on display is knows as Python Tkinter Geometry.
There are basic three Tkinter geometry
- The pack() method
- The grid() method
- The place() method
The Pack() method
Python Tkinter pack() method widget is use to organize widget in the block.
The syntax to use the pack() Method is given below
Syntax
widget.pack(options)
Example of the pack () method
from tkinter import *
parent = Tk()
redbutton = Button(parent, text = “red”, fg = “red”)
redbutton.pack( side = LEFT)
greenbutton = Button(parent, text = “Black”, fg = “black”)
greenbutton.pack( side = RIGHT )
bluebutton = Button(parent, text = “Blue”, fg = “blue”)
bluebutton.pack( side = TOP )
blackbutton = Button(parent, text = “Green”, fg = “red”)
blackbutton.pack( side = BOTTOM)
parent.mainloop()
Output of the pack () method
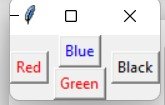
The grid() method
Python Tkinter grid() method manager organizes the widgets in the tabular form is knows as the grid () method.
The syntax to use the grid() Method is given below
Syntax
widget.grid(options)
Example of Python Tkinter grid() method
from tkinter import *
parent = Tk()
username = Label(parent,text = “UserName”).grid(row = 0, column = 0)
e1 = Entry(parent).grid(row = 0, column = 1)
password = Label(parent,text = “Password”).grid(row = 1, column = 0)
e2 = Entry(parent).grid(row = 1, column = 1)
submit = Button(parent, text = “Submit”).grid(row = 4, column = 0)
parent.mainloop()
Output Python Tkinter grid() method
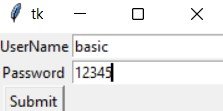
The place() method
Python Tkinter place() method manager organizes the widgets to the specific x and y coordinates is knows as Python Tkinter place() method.
The syntax to use the place() Method is given below
Syntax
widget.place(options)
Example of Python Tkinter place() method
from tkinter import *
top = Tk()
top.geometry(“400×250”)
ursername = Label(top, text = “UserName”).place(x = 30,y = 50)
email = Label(top, text = “Email”).place(x = 30, y = 90)
password = Label(top, text = “Password”).place(x = 30, y = 130)
e1 = Entry(top).place(x = 95, y = 55)
e2 = Entry(top).place(x = 75, y = 95)
e3 = Entry(top).place(x = 95, y = 135)
top.mainloop()
Output Python Tkinter place() method
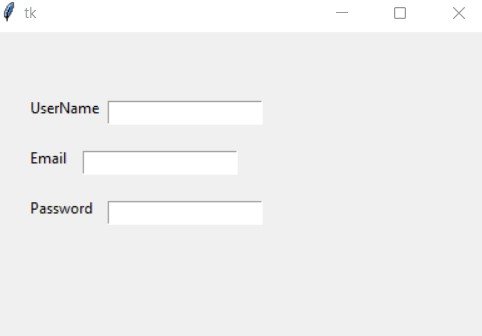
If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about Tkinter Library Function please Wikipedia click here
Stay Connected Stay Safe, Thank you.
0 Comments