Theano is a Python library that allows you to define, optimize, and efficiently evaluate mathematical expressions involve multi-dimensional arrays is knows as Theano. Theano was written at the LISA lab. It is release under a BSD license. It is mostly use in building Deep Learning Projects.
Repository :- https://github.com/Theano/Theano
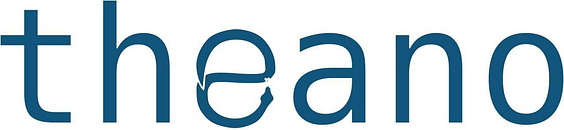
Theano features
- Tight integration with NumPy
- It is a similar interface to NumPy.
- Transparent use of a GPU
- The perform data-intensive computations up to 140x faster than on a CPU
(central Processing units). It is support for only float32.
- The perform data-intensive computations up to 140x faster than on a CPU
- Efficient symbolic differentiation
- Theano can compute derivatives for functions of one and multiple inputs.
- Speed and stability optimizations
- The avoid nasty bugs when computing expressions.
- Dynamic C code generation
- The evaluate expressions fast.
- Extensive unit-testing and self-verification
- The includes tools for detecting and diagnosing bugs.
How to install Theano
Theano can be install on Windows, MacOS, and Linux.
pip install theano
Simple Example of theano
import theano from theano import tensor s = tensor.dscalar() r = tensor.dscalar() f = s + r z = theano.function([s,r], f) y = z(2.5, 2.5) print (y)
Output of theano
5.0
Trivial Theano Expression
z = x + y
Where a, b are variables and c is the expression output.
Importing Theano
from theano import *
Declaring Variables
x = tensor.dscalar()
The dscalar method declares a decimal scalar variable. The execution of the above statement creates a variable called “x” in your program code. same, we will create variable “y” using the following statement.
y = tensor.dscalar()
Defining Expression
The define our expression that operates on these two variables “x” and “y”.
z = x + y
Defining Theano Function
s = theano.function([x,y], z)
The function takes two arguments, the first argument is input to function and the second one is its output. The above declaration states that the first argument is of type array consisting of two elements x and y. The output is a scalar unit called z. This function will be references with the variable name s in our further code.
Invoking Theano Function
r = s(4.5, 6.5)
The input to the function is an array consisting of two scalars: 4.5 and 6.5. The output of execution is assign to the scalar variable r.
print (r)
Full Program
from theano import * x = tensor.dscalar() y = tensor.dscalar() z = x + y s = theano.function([x,y], z) r = s(4.5, 6.5) print (r)
Output : 11.0
Expression for Matrix Multiplication
A dot product of two matrices. The first matrix is of dimension 2 x 3 and the second one is of dimension 3 x 2.

Declaring Variables
Theano expression for the above, we first declare two variables to represent our matrices are following:-
x = tensor.dmatrix() y = tensor.dmatrix()
Defining Expression
The dot product, we use the built-in function called dot.
z = tensor.dot(x,y)
Defining Theano Function
s = theano.function([x,y], z)
Invoking Theano Function
r= s([[ 1, 2, 3], [4, 5 ,6]], [[10, 11], [20, 21], [30,31]])
The two variables in the above statement are NumPy arrays
f(numpy.array([[ 1, 2, 3], [4, 5 ,6]]),
numpy.array([[10, 11], [20, 21], [30,31]]))
After r, we print its value
print (r)
Output
[[140. 146.] [320. 335.]]
Full program
The complete program listing is given here: from theano import * x = tensor.dmatrix() y = tensor.dmatrix() z = tensor.dot(x,y) s = theano.function([x,y], z) r = s([[ 1, 2, 3], [4, 5 ,6]], [[10, 11], [20, 21], [30,31]]) print (r)
Output
[[140. 146.] [320. 335.]]
Data Types
The different data types available to you for creating your expressions. The following table given are :-
- Byte
- 16-bit integers
- 32-bit integers
- 64-bit integers
- float
- double
- complex
Scalar
Syntax
a = theano.tensor.scalar ('a')
Example of Scalar
a = theano.tensor.scalar ('a') a = 77.0 print (a)
Output : 77.0
If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about Theano Library Function please Wikipedia click here
Stay Connected Stay Safe, Thank you.
0 Comments