In this tutorial, we will learn about the Operators in Python, or its types, and how to use them with the help of examples.
Define Operators in Python
Operators are the symbols or words those perform special operators on operands.
Python provides the following set of operator
- Arithmetic Operators
- Relational Operators or Comparison Operators
- Logical operators
- Assignment operators
- Special operators
Arithmetic operators
Arithmetic operators are use to perform mathematical operations like as addition, subtraction, multiplication and division.
Operator | Operation |
+ | Addition |
– | Subtraction |
* | Multiplication |
/ | Division |
// | Floor Division |
% | Reminder |
** | Exponent |
The Example of Arithmetic operators
Example of Arithmetic operatorsa = 81 b = 9 # addition print ('Sum: ', a + b) # subtraction print ('Subtraction: ', a - b) # multiplication print ('Multiplication: ', a * b) # division print ('Division: ', a / b) # floor division print ('Floor Division: ', a // b) # modulo print ('Modulo: ', a % b) # a to the Exponent b print ('Power: ', a ** b)
Let’s run the code
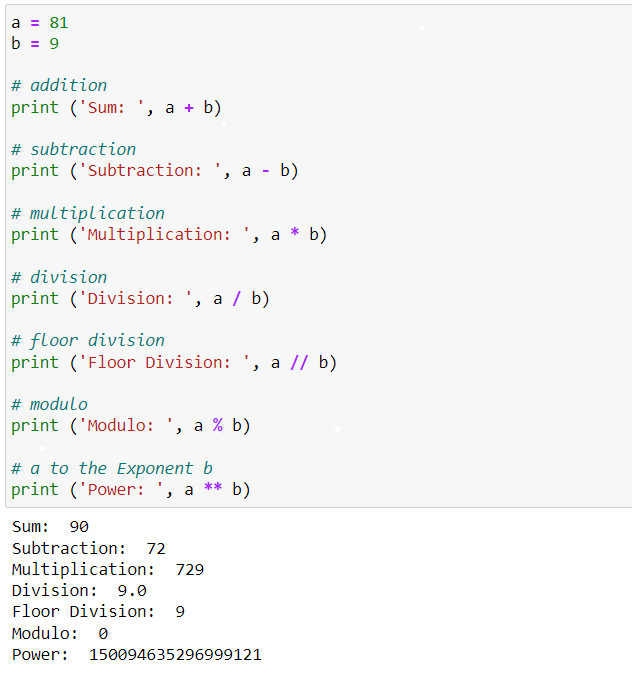
Relational Operators or Comparison Operators
This operators compares the values. It either returns True or False according to the condition is know as Relational Operators.
Chaining of relational operators is possible. In the chaining, if all comparisons returns True then only result is True. If atleast one comparison returns False then the result is False.
Operator | Description |
== | Equal To |
!= | Not Equal To |
> | Greater Than |
>= | Greater Than or Equal To |
< | Less Than |
<= | Less Than or Equal To |
The Example of Relational Operators or Comparison Operators
Example of Relational Operators or Comparison Operatorsa = 25 b = 35 # equal to operator print('a == b =', a == b) # not equal to operator print('a != b =', a != b) # greater than operator print('a > b =', a > b) # greater than or equal to operator print('a >= b =', a >= b) # less than operator print('a < b =', a < b) # less than or equal to operator print('a <= b =', a <= b)
Let’s run the code
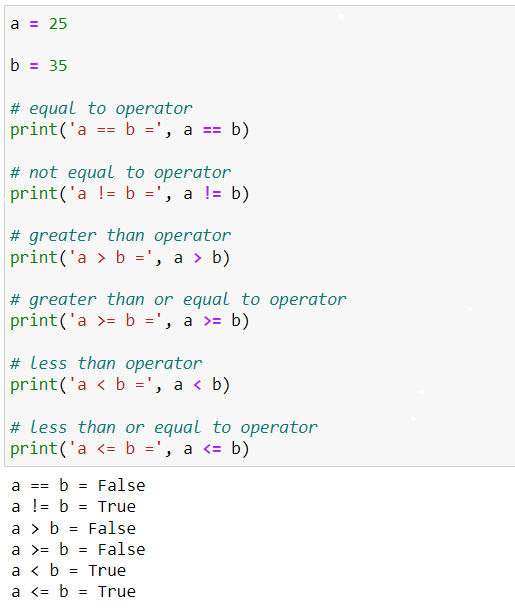
Logical operators
This operators perform Logical AND, Logical OR and Logical NOT operations.
Operator | Description |
AND | True only if both the operands are true |
OR | True if at least one of the operands is true |
NOT | True if the operand is False and vice-versa. |
The Example of Logical operators
Example of Logical operatorsa = True b = False # Print a and b is False print(a and b) # Print a or b is True print(a or b) # Print not a is False print(not a)
Let’s run the code
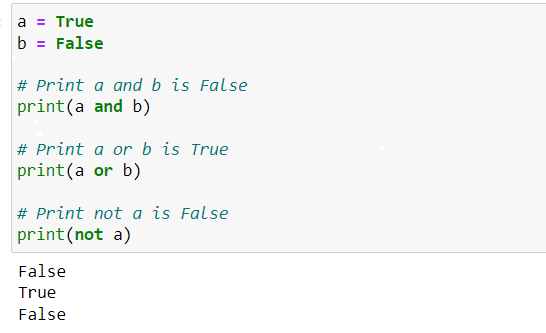
Bitwise Operators
These operators are applicable only for int and boolean types. By mistake if we are trying to apply for any other type then we will get Error.
Operator | Description |
& | Bitwise AND |
| | Bitwise OR |
– | Bitwise NOT |
^ | Bitwise XOR |
>> | Bitwise right shift |
<< | Bitwise left shift |
The Example of Bitwise Operators
Example of Bitwise Operatorsa = 81 b = 9 # Print bitwise AND operation print(a & b) # Print bitwise OR operation print(a | b) # Print bitwise NOT operation print(~a) # print bitwise XOR operation print(a ^ b) # print bitwise right shift operation print(a >> 2) # print bitwise left shift operation print(a << 2)
Let’s run the code

Assignment Operators
Operator | Description |
= | Assignment Operator |
+= | Addition Assignment |
-= | Subtraction Assignment |
*= | Multiplication Assignment |
/= | Division Assignment |
%= | Remainder Assignment |
**= | Exponent Assignment |
The Example Assignment Operators
Example Assignment Operators# Assignment Operator a = 20 # Addition Assignment a += 18 print ("a += 18 : ", a) # Subtraction Assignment a -= 8 print ("a -= 8 : ", a) # Multiplication Assignment a *= 8 print ("a *= 8 : ", a) # Division Assignment a /= 8 print ("a /= 8 : ",a) # Remainder Assignment a %= 2 print ("a %= 2 : ", a) # Exponent Assignment a **= 5 print ("a **= 5 : ", a) # Floor Division Assignment a //= 15 print ("a //= 15 : ", a)
Let’s run the code

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about Python Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments