In this tutorial, we will learn about the Multithreading in Java. How to use them with the help of examples.
Define Multithreading in Java
Multithreading is the process to achieve multitasking feature. Actually, using multithreading concept, we can execute more than one process concurrently with java program. We know every java program is a process and that may contain various sub-process. If we want to execute these sub-processes simultaneously within process or program than multithreading required.
Thread
Thread is a pre-defined class that can be use to make a class a sub-thread class by extending thread class as
Class <name> extends thread { }
Because every sub-thread class run within main thread class (Every class is a main thread class) by following time-sharing or round robin mechanism and hence its own mechanism (method) to define sub-thread class.
The creating a Threads
There are two ways to create a thread in java
- By extending thread class
- By implementing runnable interface
Lifecycle of a Thread in Java
- New
- Instance of thread create which is not yet start by invoking start().
- Runnable
- After invocation of start() & before it is select to be run by the scheduler.
- Blocked
- Thread alive, not eligible to run.
- Time Waiting
- Thread lies in a time waiting state when it calls a method with a time-out parameter.
- Terminated
- The run() method has exited.
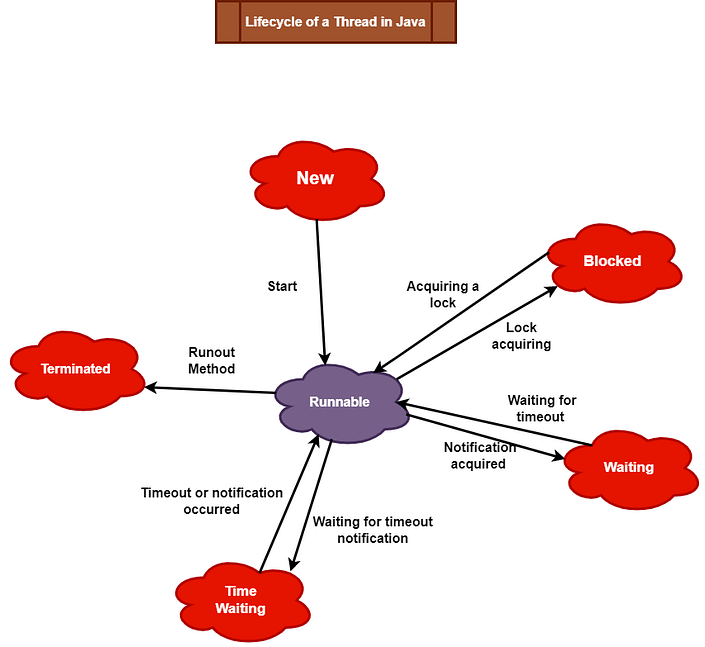
Example of Extending Thread Class
Input Value
Example of Extending Thread Classclass multi extends Thread { public void run() { try { System.out.println( "Hello Student " + Thread.currentThread().getId() + " Basic Engineer"); } catch (Exception e) { System.out.println("Exception is caught"); } } } public class theardm { public static void main(String[] args) { int n = 5; for (int i = 0; i < n; i++) { multi object = new multi(); object.start(); } } }
Let’s run the code

Output Value

Example of Implementing Runnable Interface
Example of Implementing Runnable Interfaceclass Multi implements Runnable { public void run() { try { System.out.println( "Hello Student " + Thread.currentThread().getId() + "Basic Engineer"); } catch (Exception e) { System.out.println("Company"); } } } class theardm { public static void main(String[] args) { int n = 5; for (int i = 0; i < n; i++) { Thread object = new Thread(new Multi()); object.start(); } } }

Output Value

Multi-tasking in Java
To execute more than one task or program at a time is know as Multi-tasking in Java.
Feature of Multi-tasking
- It’s Performs multiple thread execution.
- All threads share the same Memory and resource
- The CPU context switching a occurs between threads.
- It’s performance is faster when compare to Multitasking.
Types
There are two types of multi-tasking in Java
- Process-based multitasking
- More than 1 process runs concurrently.
- Thread-based multitasking
- More than 1 thread executes concurrently. A thread executes different parts of the same program.
Example of Multi-tasking
Example of Multi-taskingpublic class theardm extends Thread { public void run() { System.out.println("Basic Engineer"); } public static void main(String[] args) { theardm a = new theardm(); theardm b = new theardm(); theardm c = new theardm(); theardm d = new theardm(); a.start(); b.start(); c.start(); d.start(); } }
Let’s run the code

Output Value


Example of Multi-tasking
Input Value
Example of Multi-taskingclass key1 extends Thread { public void run() { System.out.println("Hello Student"); }} class key2 extends Thread { public void run() { System.out.println("Basic Engineer"); }} class key3 extends Thread { public void run() { System.out.println("Java tutorial"); }} class key4 extends Thread { public void run() { System.out.println("Simple Or Easy"); }} public class theardm{ public static void main(String[] args) { key1 a = new key1(); key2 b = new key2(); key3 c = new key3(); key4 d = new key4(); a.start(); b.start(); c.start(); d.start(); }}
Let’s run the code

Output Value

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about JAVA Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments