Loops in Java is use to execute a group of instructions or a block of code multiple times, without writing it repeatedly. The block of code is execute based on a certain condition.
Types of Loops in Java
Java While Loop
A while loop statement repeatedly executes a target statement as long as a given condition is true is knows as While Loop.
Flowchart
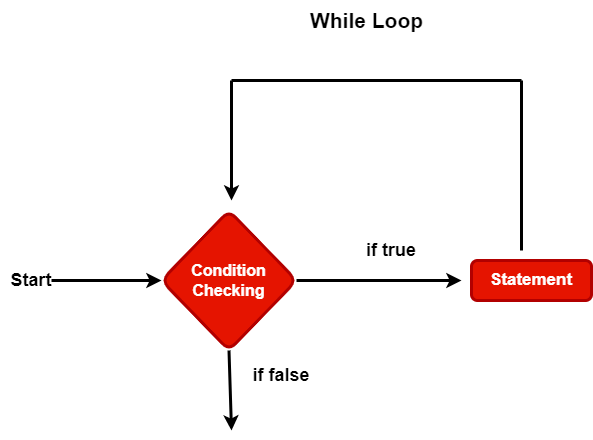
Syntax
Syntax of Java While Loopwhile (condition){ //code to be executed increment / decrement statement }
Example of while loop
Input Value
Java While Looppublic class loopw { public static void main(String[] args) { int a=0; while(a<=5){ System.out.println(a); a++; } } }
Let’s run the code
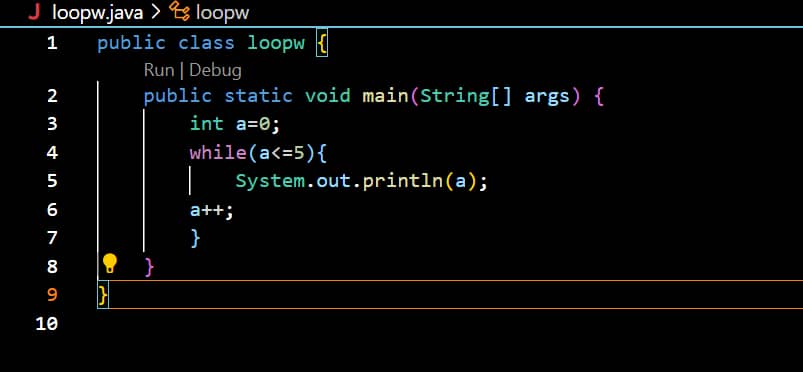
Output Value
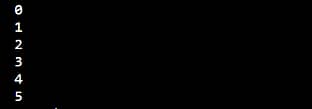
For loop in Java
For loop is the the most common use loop. It is use when we want to perform initialization, condition and increment or decrement Operation in single line.
Flowchart

Syntax
Syntax of For loop in Javafor (initialization condition; testing condition;increment/decrement) { statement(s) }
Example of For loop in Java
Input Value
For loop in Javapublic class loopw { public static void main(String[] args) { for (int a=11;a<=15;a++) { System.out.println(a); } } }
Let’s run the code

Output Value

Do While loop in Java
Do While loop is a post test loop. It is use when we want to execute loop body atleast once even condition is false. It is also knows as Exit control Loop.
Syntax
Syntax of Do While loop in Javado{ //code to be executed / loop body //update statement }while (condition);
Flowchart

Example of Do While loop in Java
Input Value
Do While loop in Javapublic class loopw { public static void main(String[] args) { int a=11; do{ System.out.println(a); a++; }while(a<=15); } }
Let’s run the code

Output Value

Enhanced for loop in Java
This feature is also include in java version 5 and later. This is special kind of for loop that can be use to access the elements of a list without giving their index number. It means it always access elements of list from start to end. It is advantageous over for loop in respect of list because it fallows two parameters only. Enhanced for loop does not replace for loop.
Syntax
Syntax of Enhanced for loop in Javafor(declaration : expression) { // Statements }
Example of Enhanced for loop in Java
Input Value
Enhanced for loop in Javapublic class loopw { public static void main(String[] args) { int [] marks = {55,66,65,57,62}; for(int a : marks ) { System.out.print( a ); System.out.print(","); } System.out.print("\n"); String [] Subject = {"HTML", "CSS", "JAVA", "c", "C++"}; for( String subject : Subject ) { System.out.print( subject ); System.out.print(","); } } }
Let’s run the code

Output Value

Labelled for loop in Java
Label is the mark in program that can be use for sending the execution control to that level.
Syntax
Syntax of Labelled for loop in Javalabelname: for(initialization; condition; incr/decr) { //functionality of the loop }
Example of Labelled for loop in Java
Input Value
Labelled for loop in Javapublic class loopw { public static void main(String[] args) { outer: for(int i = 2; i < 5; i++) { System.out.println(i); for(int j = 2; j < 4; j++) { System.out.println(j); if(i == j) continue outer; } } }}
Let’s run the code

Output Value

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about JAVA Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments