In this tutorial, we will learn about the Dictionary in Python. Its types, and how to use them with the help of examples.
List, tupple ,set data types are use to represent individual objects as a single entity. To store the group of objects as a key-value pairs use dictionary. Declare the dictionary data within the curly braces, each key separate with value by using : (colon) & each element is separate with comma. A dictionary is a data type similar to arrays, but works with keys & values instead of indexes. The keys must be unique keys but values can be duplicate. The values in the dictionary can be of any type while the keys must be immutable like numbers, tuples or strings. Dictionary keys are case sensitive- Same key name but with the different case are treat as different keys in Python dictionaries.
Note: As of Python version 3.7, dictionaries are ordered. In Python 3.6 and earlier, dictionaries are unordered.
Create a Dictionaries in Python
A Create a Dictionary in Pythonsubject = {"English": "JRR Tolkien", "Science": "Stan Lee", "History": "Edgar Allan Poe", "Math": "Roald Dahl", "Hindi": "Rabindranath Tagore"} print(subject)
Let’s run the code

In the above example, we have created a dictionary named subject. Here
- Keys are “English”, “Science”, “History”, “Math”, “Hindi”
- Value are “JRR Tolkien”, “Stan Lee”, “Edgar Allan Poe”, “Roald Dahl”, “Rabindranath Tagore”
Accessing Elements from Dictionaries in Python
The Accessing Elements from Dictionary in Pythonroll = {1: "Roshan", 2: "Soshan", 3: "Rohit", 4: "Mohit", 5: "Shivam", 6: "Shri",} print(roll[1]) print(roll[3]) print(roll[5]) print(roll[6])
Let’s run the code
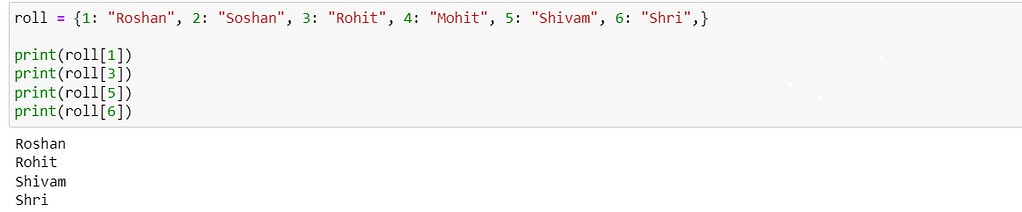
Method of Dictionary
Method | Description |
len() | Return the length (the number of items) in the dictionary. |
sorted() | Return a new sorted list of keys in the dictionary. |
clear() | Removes all items from the dictionary. |
key() | Returns a new object of the dictionary’s keys. |
value() | Returns a new object of the dictionary’s values |
all() | Return true if all keys of the dictionary are True (or if the dictionary is empty). |
any() | Return true if any key of the dictionary is true. If the dictionary is empty, return false |
items() | Returns a list of dictionary (key, value) tuple pairs |
setdefault() | Similar to get(), but will set dict[key]=default if key is not already in dict |
A Example of Dictionaries Method
Example of Dictionaries Method# clear() method dict1 = {1: "CSS", 2: "Html", 3: "JAVA", 4: "Python", 5: "c"} dict1.clear() print(dict1) # copy() method dict2 = {1: "CSS", 2: "Html", 3: "JAVA", 4: "Python", 5: "c"} demo = dict2.copy() print(demo) # pop() method dict3 = {1: "CSS", 2: "Html", 3: "JAVA", 4: "Python", 5: "c"} lo = dict3.copy() x = lo.pop(2) print(x) # keys() method dict4 = {1: "CSS", 2: "Html", 3: "JAVA", 4: "Python", 5: "c"} print(lo.keys()) # popitem() method dict5 ={1: "CSS", 2: "Html", 3: "JAVA", 4: "Python", 5: "c"} dict_demo.popitem() print(dict_demo)
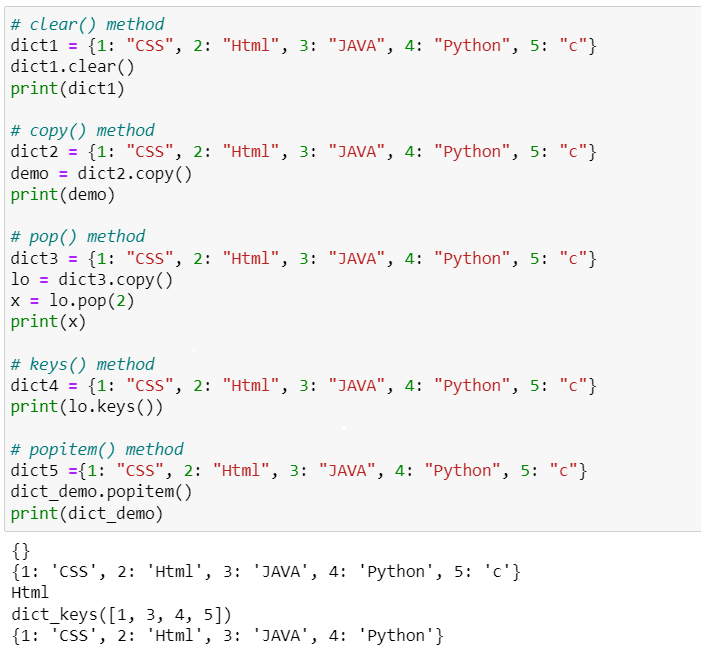
Nested Dictionaries
A dictionary within another dictionary is know as dictionary or nesting of a dictionary.

A Nested DictionaryDict = {1: 'Basic', 2: 'Engineer', 3: {'A': 'Hello', 'B': 'Student', 'C': 'Python'}} print(Dict)

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about Python Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments