Structure in c is a user define data type that enables us to store the collection of different data types. Structures simulate the use of classes and templates as it can store various information. The struct keyword is use to define the structure is knows as Structure.
Syntax
- struct structure_name
- {
- data_type member1;
- data_type member2;
- .
- .
- data_type memeberN;
- };
Accessing Structure Members
To access any member of a structure, we use the member access Dot (.) operator. The member access operator is code as a period between the structure variable name and the structure member that we wish to access.
Example
Input value
#include <stdio.h> #include <string.h> struct copy { char title[50]; char author[50]; char subject[100]; int copy_id; }; int main( ) { struct copy copy1; struct copy copy2; strcpy( copy1.title, "HTML"); strcpy( copy1.author, "Neeraj"); strcpy( copy1.subject, "basic engineer"); copy1.copy_id = 524163; strcpy( copy2.title, "CSS"); strcpy( copy2.author, "Rohit "); strcpy( copy2.subject, "basic engineer"); copy2.copy_id = 4556421; printf( "copy 1 title : %s\n", copy1.title); printf( "copy 1 author : %s\n", copy1.author); printf( "copy 1 subject : %s\n", copy1.subject); printf( "copy 1 copy_id : %d\n", copy1.copy_id); printf( "copy 2 title : %s\n", copy2.title); printf( "copy 2 author : %s\n", copy2.author); printf( "copy 2 subject : %s\n", copy2.subject); printf( "copy 2 copy_id : %d\n", copy2.copy_id); return 0; }
Output value

Structures as Function Arguments
Pass a structure as a function argument in the same way as you pass any other variable or pointer.
Example
Input value
#include <stdio.h> #include <string.h> struct Books { char title[30]; char author[40]; char subject[80]; int book_id; }; void printBook( struct Books book ); int main( ) { struct Books Book1; struct Books Book2; strcpy( Book1.title, "HTML"); strcpy( Book1.author, "Neeraj"); strcpy( Book1.subject, "baise engineer"); Book1.book_id = 5897; strcpy( Book2.title, "CSS"); strcpy( Book2.author, "Rohit"); strcpy( Book2.subject, "baise engineer"); Book2.book_id = 45865; printBook( Book1 ); printBook( Book2 ); return 0; } void printBook( struct Books book ) { printf( "Book title : %s\n", book.title); printf( "Book author : %s\n", book.author); printf( "Book subject : %s\n", book.subject); printf( "Book book_id : %d\n", book.book_id); }
Output value

Pointers to Structures
Pointers to structures in the same way as you define pointer to any other variable
Syntax
struct Books *struct_pointer;
Example
Input value
#include <stdio.h> #include <string.h> struct Books { char title[40]; char author[45]; char subject[90]; int book_id; }; void printBook( struct Books *book ); int main( ) { struct Books Book1; struct Books Book2; strcpy( Book1.title, "HTML"); strcpy( Book1.author, "Neeraj"); strcpy( Book1.subject, "Basic engineer"); Book1.book_id =52525; strcpy( Book2.title, "CSS"); strcpy( Book2.author, "Rohit"); strcpy( Book2.subject, "Basic engineer"); Book2.book_id =85214; printBook( &Book1 ); printBook( &Book2 ); return 0; } void printBook( struct Books *book ) { printf( "Book title : %s\n", book->title); printf( "Book author : %s\n", book->author); printf( "Book subject : %s\n", book->subject); printf( "Book book_id : %d\n", book->book_id); }
Output Value
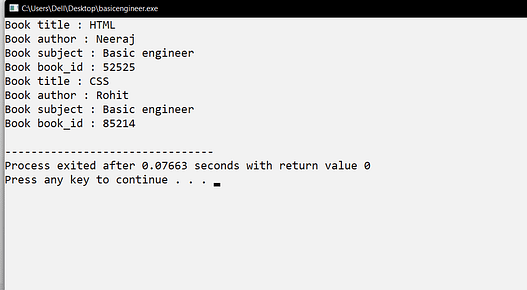
typedef in C
The typedef is a keyword use in C programming language. typedef keyword is used to redefine the name of an already existing variable.
Syntax of typedef
typedef <existing_name> <alias_name>
Eaxmple
Input Value
#include <stdio.h> int main() { typedef unsigned int unit; unit a,b; a=45; b=52; printf("Value of a :%d",a); printf("\nValue of b :%d",b); return 0; }
Output Value

Array of Structures in C
The collection of multiple structures variables where each variable contains information about different entities. The array of structures in C are use to store information about multiple entities of different data types.
Example
Input value
#include<stdio.h> #include <string.h> struct student{ int rollno; char name[25]; }; int main(){ int i; struct student st[3]; printf("Enter Records of 3 students"); for(i=0;i<3;i++){ printf("\nEnter Rollno:"); scanf("%d",&st[i].rollno); printf("\nEnter Name:"); scanf("%s",&st[i].name); } printf("\nStudent Information List:"); for(i=0;i<3;i++){ printf("\nRollno:%d, Name:%s",st[i].rollno,st[i].name); } return 0; }
Output value

Nested Structure in C
A nested structure in C is a structure within structure. One structure can be declares inside another structure in the same way structure members are declare inside a structure is knows as Nested Structure in c.
Example
Input value
#include <stdio.h> #include <string.h> struct Employee { int id; char name[45]; struct Date { int dd; int mm; int yyyy; }DOB; }e1; int main( ) { e1.id=25; strcpy(e1.name, "Rohit Kumar"); e1.DOB.dd=25; e1.DOB.mm=12; e1.DOB.yyyy=2022; printf( "employee id : %d\n", e1.id); printf( "employee name : %s\n", e1.name); printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.DOB.dd,e1.DOB.mm,e1.DOB.yyyy); return 0; }
Output value

Union
It is a user-define data type which is a collection of different variables of different data types in the same memory location. The union can also be define as many members, but only one member can contain a value at a particular point in time is knows as Union.
Syntax
union [union tag] { member definition; member definition; ... member definition; } [one or more union variables];
Example
Input Value
#include <stdio.h> #include <string.h> union Data { int a; float b; char c[20]; }; int main( ) { union Data data; data.a = 25; data.b= 52.3; strcpy( data.c ,"HTML"); printf( "data.a: %d\n", data.a); printf( "data.b: %f\n", data.b); printf( "data.c: %s\n", data.c); return 0; }
Output Value

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about C Programming language please Wikipedia click here .
Stay Connected Stay Safe, Thank you
0 Comments