String in C programming is a sequence of characters with a null character ‘\0’. Strings are defines as an array of characters is knows as Strings. Strings are actually one-dimensional array.
Declare
There are two ways to declare a string in c language.
- By char array
- By string literal
The example of declaring string by char array in C language.
char ch[12]={‘b’, ‘a’, ‘s’, ‘i’, ‘c’, ‘e’, ‘n’, ‘g’, ‘i’, ‘e’, ‘e’, ‘r’, ‘\0’};
Array index starts from 0, so it will be represented as in the figure

String Example in C
Input Value
#include<stdio.h> #include <string.h> int main(){ char h[12]={'b', 'a', 's', 'i', 'c', 'e', 'n', 'g', 'i', 'e', 'e', 'r', '\0'}; char r[12]="basicengieer"; printf("Char Array : %s\n", h); printf("String Literal : %s\n", r); return 0; }
Output Value
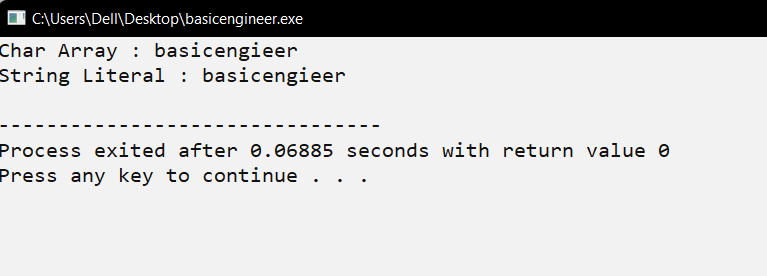
Traversing String
Traversing the string is one of the most important aspects in any of the C programming language. It is somewhat different from the traversing an integer array.
There are two ways to traverse a string
- Using the length of string.
- Using the null character.
Using the length of string
Example
input value
#include<stdio.h> void main () { char s[13] = "Basicengieer"; int i = 0; int count = 0; while(i<13) { if(s[i]=='a' || s[i] == 'i' || s[i] == 'e' || s[i] == 'i' || s[i] == 'e' ||s[i] == 'e') { count ++; } i++; } printf("The number of vowels %d",count); }
Output value
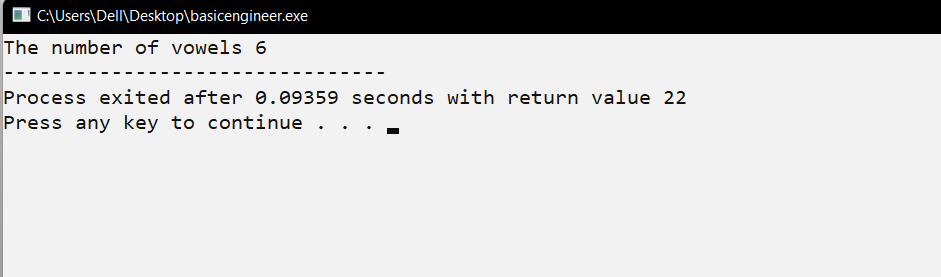
Using the null character
Example
Input value
#include<stdio.h> void main () { char s[13] = "Basicengieer"; int i = 0; int count = 0; while(s[i] != NULL) { if(s[i]=='a' || s[i] == 'i' || s[i] == 'e' || s[i] == 'i' || s[i] == 'e' ||s[i] == 'e') { count ++; } i++; } printf("Number of vowels %d",count); }
Output Value
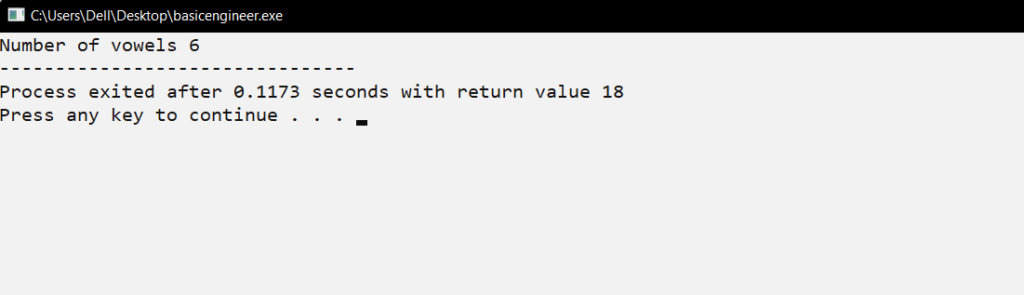
C gets() and puts() functions
It is declare in the header file stdio.h. Both the functions are involve in the i/o operations of the strings.
Gets() function
gets() function is define in the <stdio.h> header file of C. C library also facilitate a special function to read a string from a user is knows as Get() function. This function is represent as gets() function.
Syntax
- char[] gets(char[]);
Example
Input value
#include <stdio.h> int main () { char rom[20]; printf("Enter a string : "); gets(rom); printf("You enter: %s", rom); return(0); }
Output Value
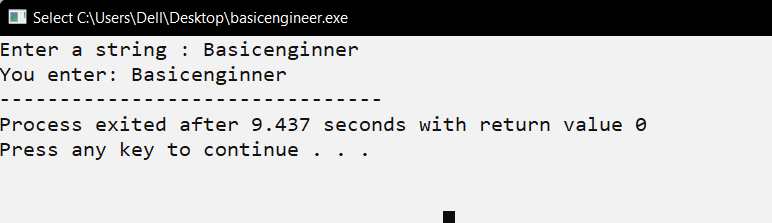
Puts() Function
puts() function is define in the <stdio.h> header file of C. C library also facilitate a special function to print a string on the console screen is knows as puts() function. This function is represent as puts() function.
Syntax
- int puts(char[]);
Example
Input value
#include<stdio.h> #include <string.h> int main(){ char string[50]; printf("Enter a string: "); gets(string); printf("you enter: "); puts(string); return 0; }
Output Value

C String Functions
Function | Description |
strcpy | The copy the source string to destination string. |
strcat | It is a concatenates first string with second string. |
strlen | The returns the length of string name. |
strcmp | It is a compares the first string with second string. |
strlwr | String characters in lowercase. |
strupr | String characters in Uppercase. |
strcpy Function
Example
Input value
#include<stdio.h> #include <string.h> int main(){ char r[15]={'b', 'a', 's', 'i', 'c', 'e', 'n', 'g', 'i', 'e', 'e', 'r', '\0'}; char t[15]; strcpy(t,r); printf("second string : %s",t); return 0; }
Output value

strcat Function
Example
Input value
#include<stdio.h> #include <string.h> int main(){ char y[15]={'b', 'a', 's', 'i', 'c', 'e', 'n', 'g', 'i', 'e', 'e', 'r', '\0'}; char u[15]={'h', 'e', 'l', 'l', 'o', '\0'}; strcat(y,u); printf("Value of first string is: %s",y); return 0; }
Output value

strlen Function
Example
Input value
#include<stdio.h> #include <string.h> int main(){ char ch[20]={'b', 'a', 's', 'i', 'c', 'e', 'n', 'g', 'i', 'e', 'e', 'r', '\0'}; printf("Length of string is: %d",strlen(ch)); return 0; }
Output value
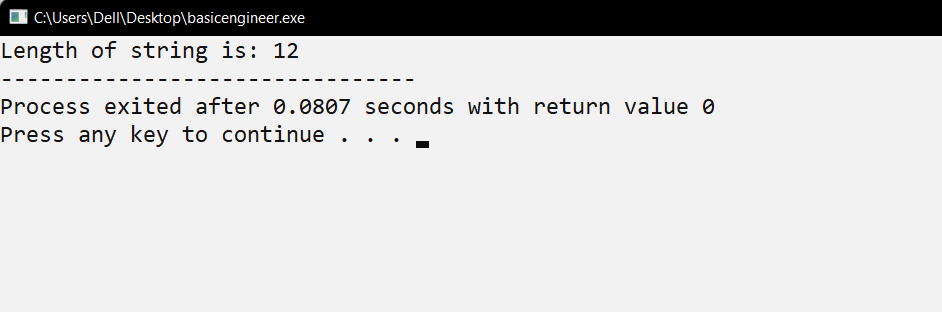
strcmp Function
Example
Input value
Output value
unequal Output value
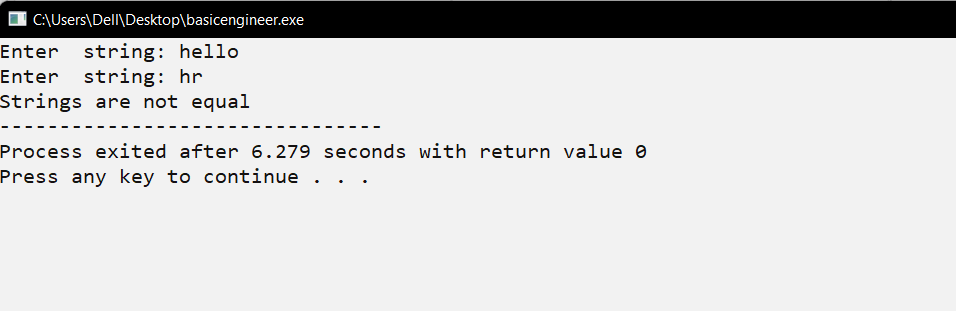
Equal output value
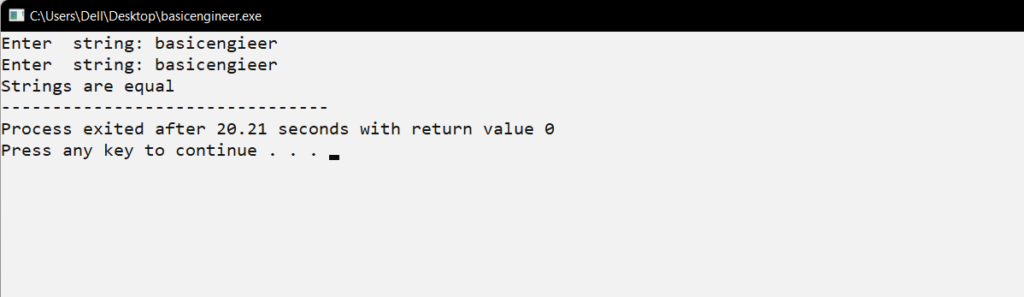
strlwr
Example
Input value
#include<stdio.h> #include <string.h> int main(){ char rom[52]; printf("Enter string: "); gets(rom); printf("\nLower String is: %s",strlwr(rom)); return 0; }
Output value

strupr Function
Example
Input value
#include<stdio.h> #include <string.h> int main(){ char rom[52]; printf("Enter string: "); gets(rom); printf("\nUpperCase String is: %s",strupr(rom)); return 0; }
Output value
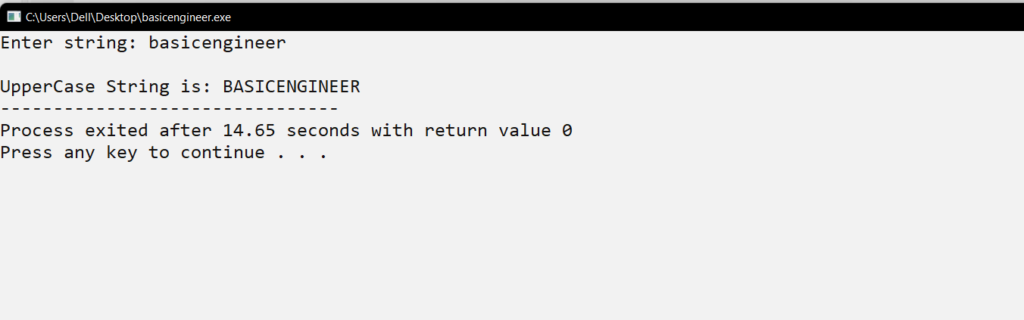
strrev Function
Example
Input Value
#include<stdio.h> #include <string.h> int main(){ char x[58]; printf("Enter string: "); gets(x); printf("\nReverse String is: %s",strrev(x)); return 0; }
Output value

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about C Programming language please Wikipedia click here .
Stay Connected Stay Safe, Thank you
0 Comments