NumPy is stand for “Numerical Python”. it is a Python library. NumPy is use for works with arrays. It is provides support in mathematical, scientific, engineering, and data science programming. NumPy is an open-source library in Python. Numpy was create by Travis Oliphan in 2005.
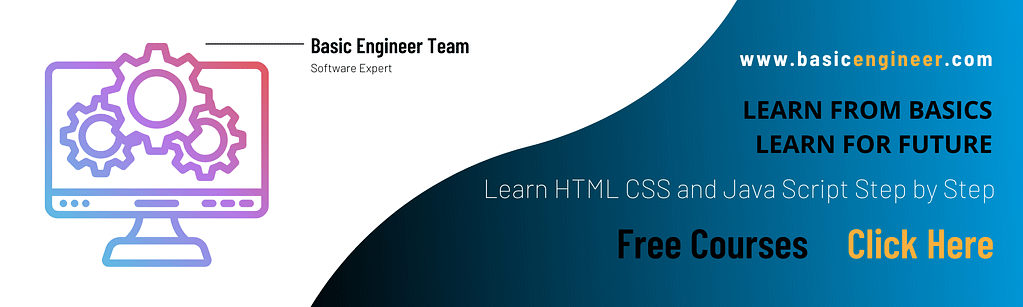
I am going to explain in a very basic approach, and you will thank me later after reading this small capsule sharing with you here. It will really help you to understand better.
Creating a Numpy Array
Numpy can be creates by multiple ways, with various number of Ranks, defining the size of the Array. Arrays can also be creates with the use of various data types is knows Creating a Numpy Array.
Create a NumPy ndarray Object
import numpy as np
what = np.array((0,1,2,3,4,5))
print(what)
Output: 0,1,2,3,4,5.
Dimensions of Arrays
four types of Dimension of Arrays
- 0-D Arrays
- 1-D Arrays
- 2-D Arrays
- 3-D Arrays
0-D Arrays
0-D arrays are the elements in array. Each value in array is 0-D array is knows as 0-D Array.
Example of 0-D
import numpy as np
what = np.array(5)
print(what)
Output : 5
1-D Arrays
Array that has 0-D arrays as its elements is knows as 1-D array.
Example of 1-D Array
import numpy as np
what = np.array((0,1,2,3,4,5))
print(what)
Output : [0 1 2 3 4 5]
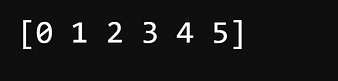
2-D Array
Array that has 1-D arrays as its elements is knows as 2-D array.
Example of 2-D Array
import numpy as np
what = np.array([[0, 1, 3],[9, 10, 11]])
print(what)
Output: [[ 0 1 3] [ 9 10 11]]
3-D Array
Array that has 2-D arrays as its elements is knows as 3-D array.
Example of 3-D Array
import numpy as np
what = np.array([[[0, 1, 2], [3, 4, 5]], [[2, 4, 6], [8, 10, 12]]])
print(what)
Output: [[[ 0 1 2 ][3 4 5 ]][[2 4 6 ][8 10 12 ]]]
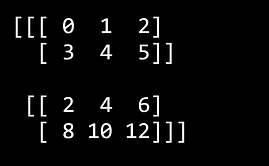
Indexing Array
Indexing can be done in numpy by using an array as an index. Numpy arrays can be index with other arrays or any other sequence with the exception of tuples is knows as Indexing Array.
Example of Indexing Array
import numpy as np
what = np.array([1,2,3,4,5,6])
print(what[3])
Output: 4
Other Example of Indexing Array
import numpy as np
what = np.array([1,2,3,4,5,6])
print(what[3] + what[5])
Output: 10
Slicing Array
Slicing means taking elements from one given index to another given index is knows as Slicing Array.
Example of Slicing Array
import numpy as np
what = np.array([11, 12,13,14,15,16,17])
print(what[2:5])
Output: [13 14 15 ]
Other Example of Slicing Array
import numpy as np
what = np.array([11, 12,13,14,15,16,17])
print(what[2:])
Output: [13 14 15 ]
Other Example of Slicing Array
import numpy as np
what = np.array([11, 12,13,14,15,16,17])
print(what[:2])
Output: [11 12 13 ]
Datatypes
Every ndarray has an associates data type object. Some Datatypes
- i – integer
- b – boolean
- f – float
- s – string
- o – object
- u – unsigned integer
- c – complex float
- m – timedelta
- M – datetime
- U – unicode string
Check the Data Type Array
Example of Data Type Array
import numpy as np
what = np.array([0,1,2,3])
print(what.dtype)
Output: int64
Create an array with data type
Example
import numpy as np
arr = np.array([11, 12, 13, 14], dtype=’i’)
print(arr)
print(arr.dtype)
Output : [11 12 13] int32
numpy.reshape()
The numpy.reshape() function is available in NumPy package. The shape of an array is the number of elements in each dimension.
Example of numpy.reshape()
import numpy as np
what = np.array([[11, 12, 13, 14], [15, 16, 17, 18]])
print(what.shape)
Output: (2,4)
NumPy Array Reshaping
The numpy.reshaping() function is available in NumPy package. reshape means is a ‘changes in shape’ is knows as reshaping
Example of NumPy Array Reshaping
Reshape From 1-D to 2-D
import numpy as np
what = np.array([1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16])
newwhat = what.reshape(4, 4)
print(newwhat)
Output:
[[ 1 2 3 4] [ 5 6 7 8] [ 9 10 11 12] [13 14 15 16]]
Example of NumPy Array Reshaping
Reshape From 1-D to 3-D
import numpy as np
what = np.array([11,12,13,14,15,16,17,18,19,20,21,22])
newwhat = what.reshape(2, 3, 2)
print(newwhat)
Output
[[[11 12] [13 14] [15 16]] [[17 18] [19 20] [21 22]]]
Iterating Array
Iterating Array is a means going through elements one by one. It is a multi-dimensional arrays in numpy.
Example of Iterating Array
import numpy as np
what = np.array([4,5,6])
for y in what:
print(y)
Output:
4 5 6
Join NumPy Arrays
Exmaple
import numpy as np
what = np.array([4, 5, 6])
why = np.array([8,7,9])
where = np.concatenate((what, why))
print(where)
Output
[4 5 6 8 7 9]
Split NumPy Arrays
Example of Splitting NumPy Arrays
import numpy as np
what = np.array([4, 5, 6, 7, 8, 9])
newwhat = np.array_split(what, 2)
print(newwhat)
Output:
[array([4, 5, 6]), array([7, 8, 9])]
If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about pandas please Wikipedia click here
0 Comments