Maths Functions in Java are define within Math class. The most of math functions are static functions that’s why used always will Math class as Math.method();.
Methods of Maths Functions in Java
sqrt()
Returns square root value
Syntax
Syntax of sqrt()double Math.sqrt(double)
Example of sqrt()
Input Value
sqrt()public class mathsm { public static void main(String[] args) { //sqrt double x = 9.0; System.out.println(Math.sqrt(x)); } }
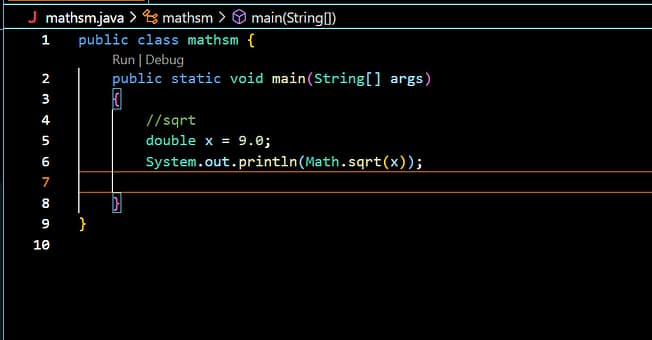
Output Value

pow()
Returns power value of a number raised to some power
Syntax
Syntax of pow()double Math.pow(double,double);
Example
Input Value
pow()public class mathsm { public static void main(String[] args) { double x = 2; double y = 16; System.out.println(Math.pow(x, y)); } }
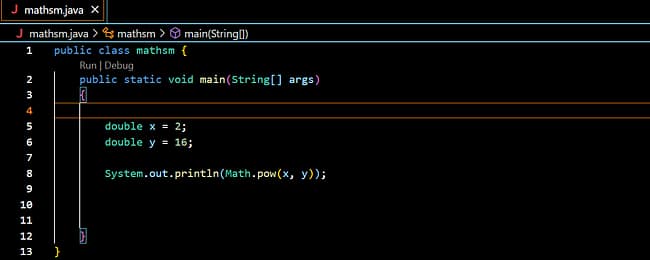
Output Value

sin()
Returns sin value
Syntax
Syntax of sin()double
Example
Input Value
sin()public class mathsm { public static void main(String[] args) { double x = 30; double y = Math.toRadians(x); System.out.println(Math.sin(y)); } }

Output Value

exp()
Return exponent value
Syntax
Syntax of exp()double Math.exp(double)
Example
Input Value
exp()public class mathsm { public static void main(String[] args) { double x = 4; System.out.println(Math.exp(x)); } }

Output Value

log()
Returns log value whose base is e.
Syntax
Syntax of log()double Math.log(double)
Example
Input Value
log()public class mathsm { public static void main(String[] args) { double x = 81.23; System.out.println(Math.log(x)); } }

Output Value

Floor()
Return nearest down integer of the given factorial value
Syntax
Syntax of Floor()Math.floor(factorial value);
Example
Input Value
Floor()public class mathsm { public static void main(String[] args) { double a = 12.2574; System.out.println(Math.floor(a)); } }

Output Value

Ceil()
This method returns nearest up integer value of the given fraction value
Syntax
Syntax of Ceil()math.ceil(function);
Example
Input Value
Ceil()public class mathsm { public static void main(String[] args) { double y = 12.369852147; System.out.println(Math.ceil(y)); } }

Output Value

round()
This function rounds off the decimal post digits of the given fractional number. It will work as suppose there is one digit after decimal in fractional number and if it is 5 or greater than 5 then it will be treat as 10 otherwise 0, and so on for two ,three…. digits after decimal position.
Syntax
Syntax of round()Math.round (fractional number);
Example
Input Value
round()public class mathsm { public static void main(String[] args) { double y = 12.36; System.out.println(Math.round(y)); } }

Output Value

abs()
this function returns the positive value of the given negative value but vice-versa not true.
Syntax
Syntax of abs()public static int abs(int i) public static double abs(double d) public static float abs(float f) public static long abs(long lng)
Example
Input Value
abs()public class mathsm { public static void main(String[] args) { float x = -23.5f; long y = 255525; double z = 258.3674; int r = 12; System.out.println(Math.abs(x)); System.out.println(Math.abs(y)); System.out.println(Math.abs(z)); System.out.println(Math.abs(r)); } }

Output Value

max()
This function returns the maximum number of the given two numbers. Numbers array of any numeric type.
Syntax
Syntax of max()Math.max(value1,value2);
Example
Input Value
max()public class mathsm { public static void main(String[] args) { int x = 25; int y = 58; System.out.println(Math.max(x, y)); } }

Output Value

min()
This function returns the minimum number of the given two numbers. Numbers array of any numeric type.
Syntax
Syntax of min()Math.min(value1,value2);
Example
Input Value
min()public class mathsm { public static void main(String[] args) { int x = 25; int y = 58; System.out.println(Math.min(x, y)); } }

Output Value

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about JAVA Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments