Decision making in Java structures have one or more conditions to be evaluate or test by the program, along with a statement or statements that are to be execute if the condition is determine to be true, and optionally, other statements to be execute if the condition is determine to be false.
There are six Selection statements in Java.
- If statement
- If-else statement
- If-else-if ladder
- Nested if statement
- Switch statement
- Jump statement
If Statement
The Java if statement tests the condition. It executes the if block if condition is true.
Flow Chart
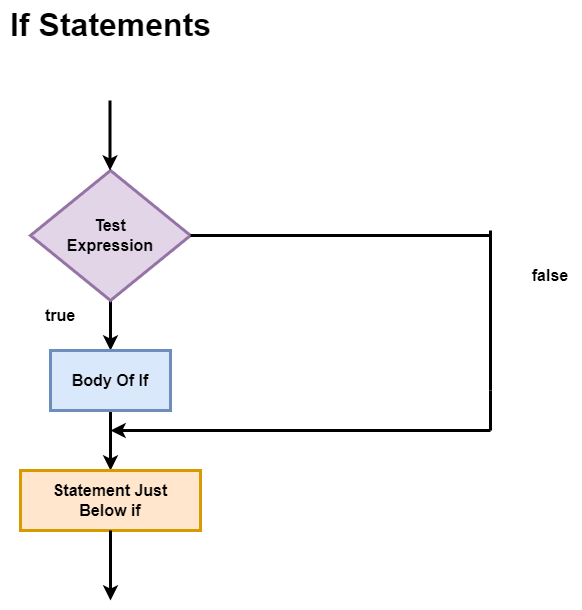
Syntax
If Statement in Javaif(condition) { // Statements to execute if // condition is true }
Example of If Statement
Input Value
If Statement in Javapublic class ifv { public static void main(String[] args) { int age=56; if(age>25){ System.out.print("Age is greater than 25"); } } }
Let’s run the code
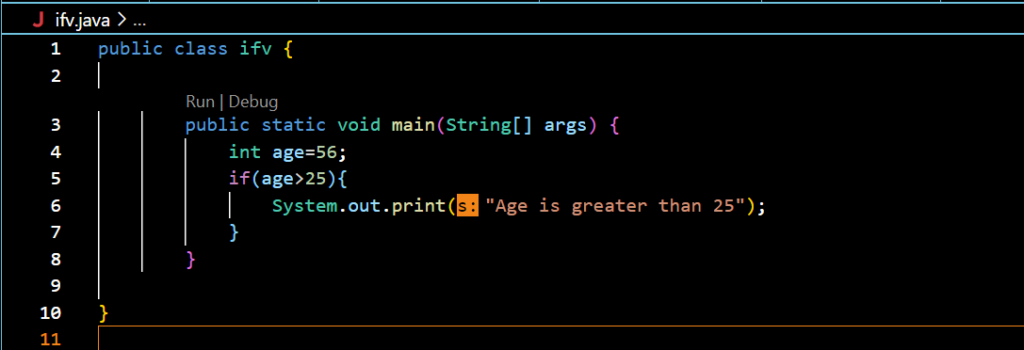
Output Value

If-else Statement
The Java if-else statement also tests the condition. It executes the if block if condition is true otherwise else block is executes.
Flowchart
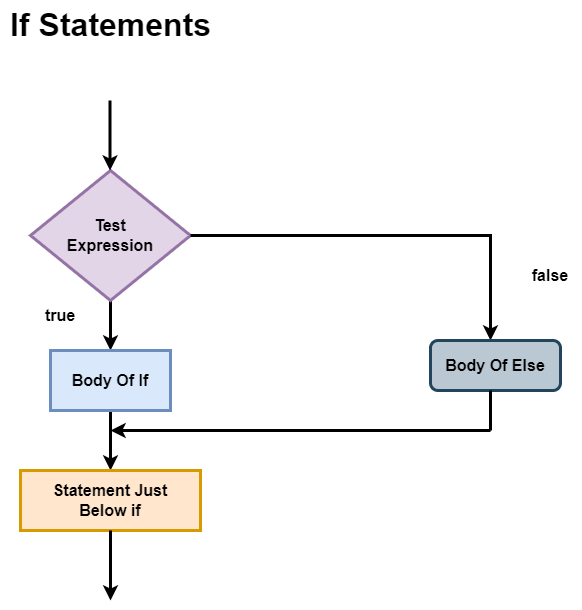
Syntax
If-else Statement in Javaif (condition) { // Executes this block if // condition is true } else { // Executes this block if // condition is false }
Example of If Statement
Input Value
If-else Statement in Javapublic class elsev { public static void main(String[] args) { int number=60; if(number%2==0){ System.out.println("even number"); }else{ System.out.println("odd number"); } } }
Let’s run the code
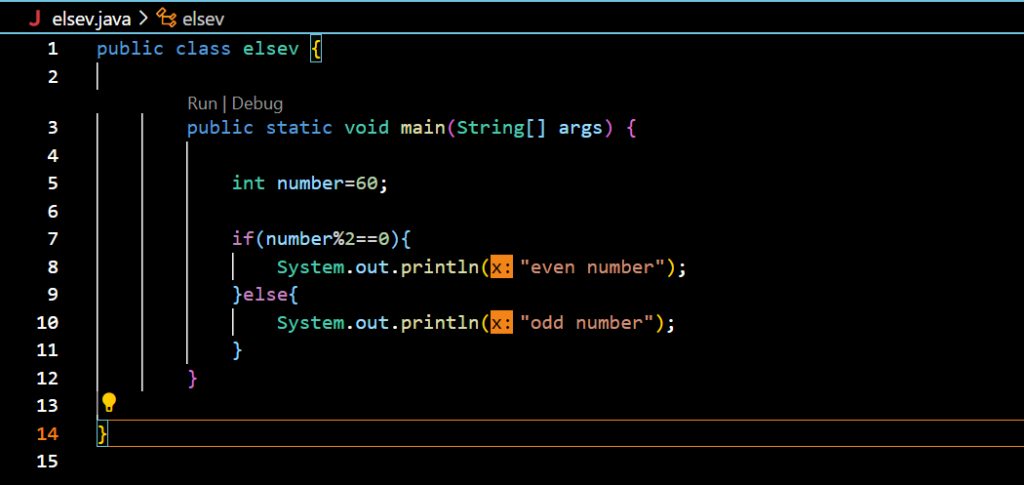
Output Value

If-else-if ladder
The if-else-if ladder statement executes one condition from multiple statements.
Flowchart
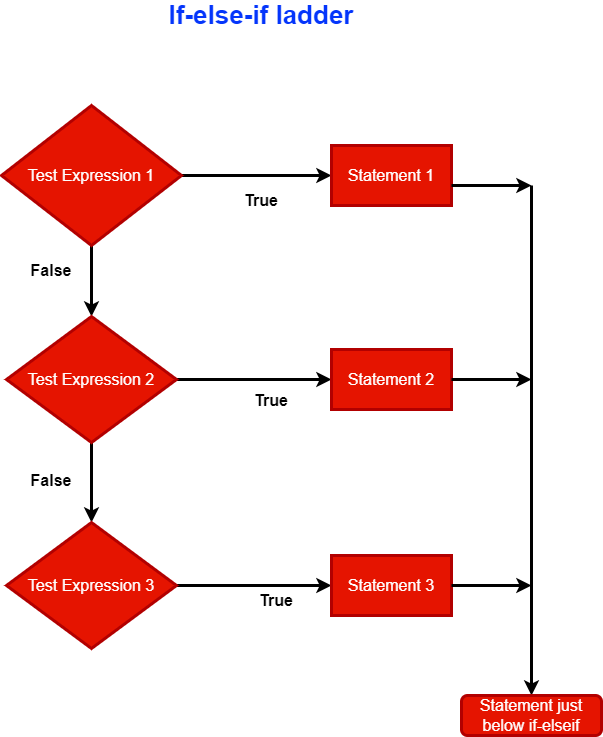
Syntax
If-else-if ladderif (condition) statement; else if (condition) statement; . . else statement;
Example of If Statement
Input Value
If-else-if ladderspublic class elseifv { public static void main(String[] args) { int marks=85; if(marks<40){ System.out.println("fail"); } else if(marks>=45 && marks<60){ System.out.println("D grade"); } else if(marks>=62 && marks<72){ System.out.println("C grade"); } else if(marks>=72 && marks<82){ System.out.println("B grade"); } else if(marks>=82 && marks<90){ System.out.println("A grade"); }else if(marks>=90 && marks<100){ System.out.println("A+ grade"); }else{ System.out.println("Invalid!"); } } }
Let’s run the code
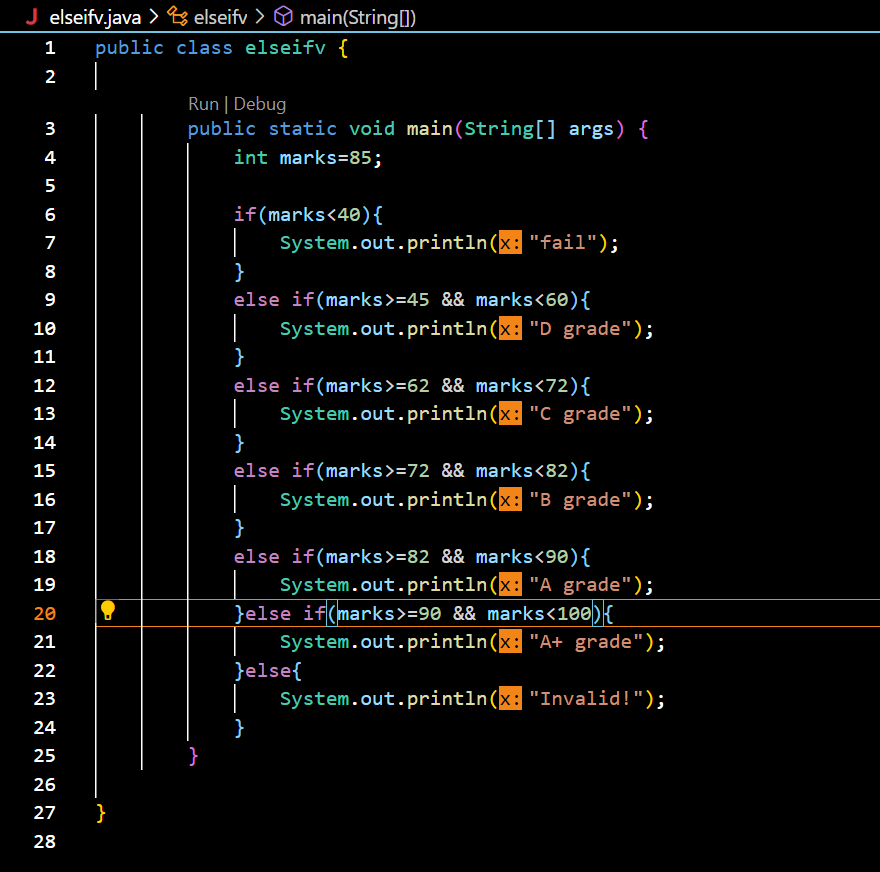
Output Value

Nested if Statement
The nested if statement represents the if block within another if block. Here, the inner if block condition executes only when outer if block condition is true.
Flowchart
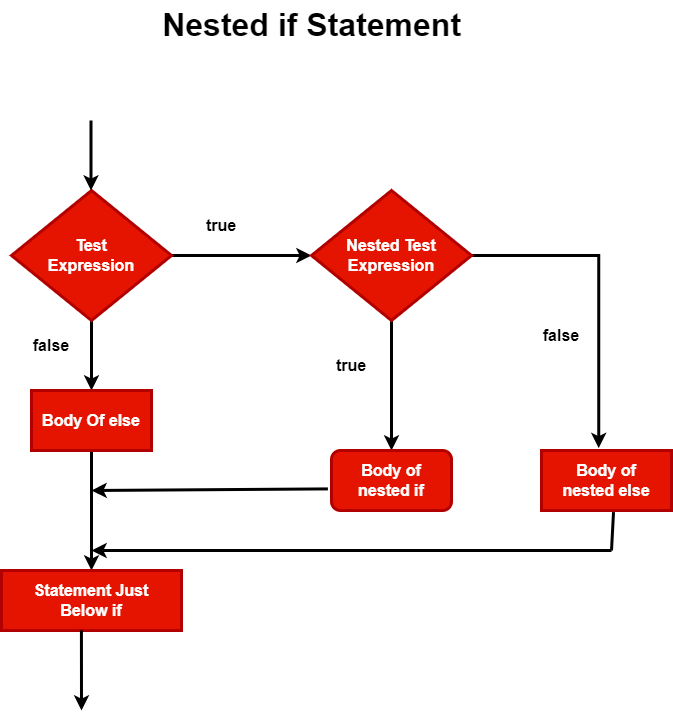
Syntax
Nested if Statement in Javaif (condition1) { // Executes when condition1 is true if (condition2) { // Executes when condition2 is true } }
Example of If Statement
Input Value
Nested if Statement in Javapublic class neastedv { public static void main(String[] args) { int age=30; int weight=80; if(age>=25){ if(weight>50){ System.out.println("You are eligible to donate blood"); } } } }
Let’s run the code
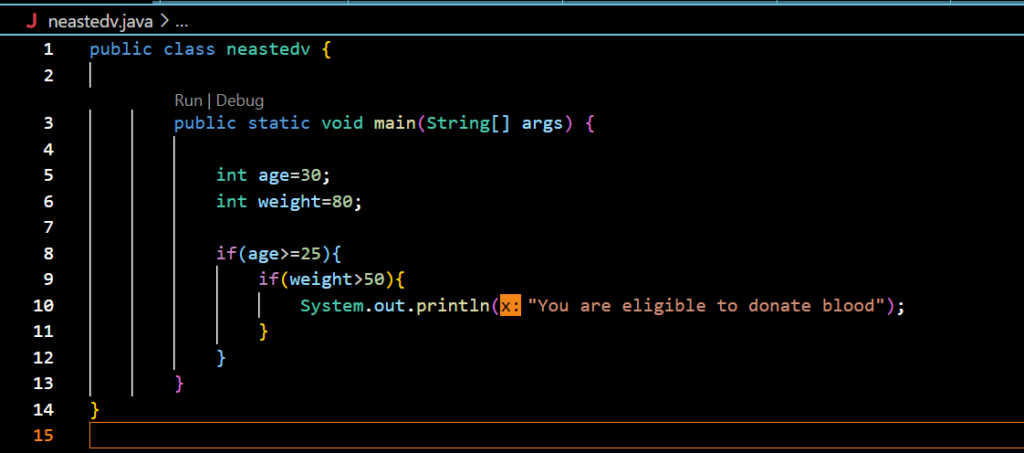
Output Value

Switch Statement
Syntax
Syntax of Switch Statementswitch (expression) { case value1: statement1; break; case value2: statement2; break; . . case valueN: statementN; break; default: statementDefault; }
Example of If Statement
Input Value
Switch Statementpublic class arrays { public static void main(String[] args) { int day = 2; String dayString; switch (day) { case 1: dayString = "Monday"; break; case 2: dayString = "Tuesday"; break; case 3: dayString = "Wednesday"; break; case 4: dayString = "Thursday"; break; case 5: dayString = "Friday"; break; case 6: dayString = "Saturday"; break; case 7: dayString = "Sunday"; break; default: dayString = "Invalid"; } System.out.println(dayString); } }
Let’s run the code
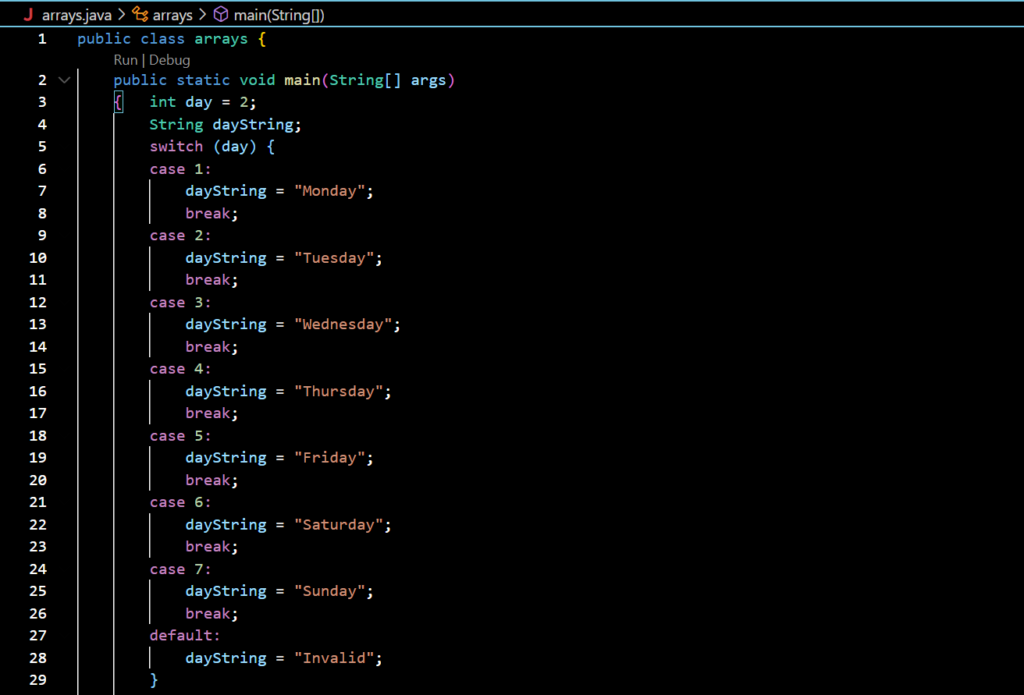
Output Value

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about JAVA Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments