Data types are different sizes and values that can be stored in the variable is knows as Data types in Java Programming.
Types
There are two types of Data Types in Java
- Primitive data types
- Non-primitive data types
Primitive Data Types in JAVA
Primitive data type specifies the size and type of variable values. It has no additional methods is knows as Primitive Data Types.
There are Eight types of Primitive Data types
- Boolean data type
- Byte data type
- Char data type
- Short data type
- Int data type
- Long data type
- Float data type
- Double data type
Boolean Data Types
Boolean data type represents only one bit of information either true or false values.
Size: 1 bit
Values: true or false
Default Value: false
Syntax
boolean booleanVar;
Example of Boolean Data Types
Input Value
Boolean Data Typespublic class bool { public static void main(String[] args) { int x = 20; System.out.println(x == 15); System.out.println(x == 20); System.out.println(x < 35); System.out.println(x >= 10); System.out.println(x <= 15); } }
Let’s Run the code
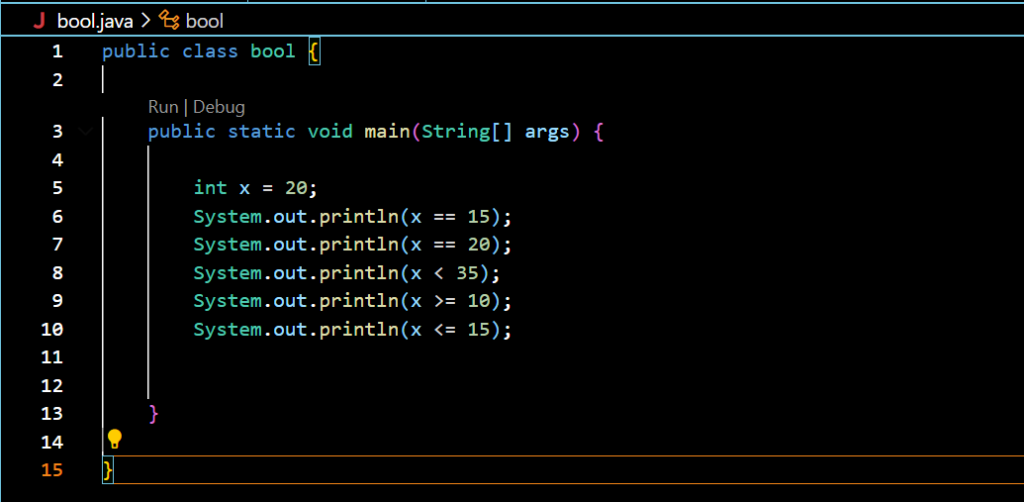
Output Values

Byte data type
The byte data type is an 8-bit signed two’s complement integer.
Size: 1 byte or (8 bit)
Values: -128 to 127
Default Value: 0
Syntax
byte byteVar;
Example of Byte data type
Input Value
Byte data typepublic class bytevariable{ public static void main(String[] args) { byte a = 15; byte b = 25; System.out.println("The sum of variable is :"+ (a+b)); } }
Let’s Run the code


Char data type
This data type is a single 16-bit Unicode character.
Size: 2 byte or (16 bits)
Values: ‘\u0000’ or (0) to ‘\uffff’ (65535)
Default Value: ‘\u0000’
Syntax
char charVar;
Example Char data type
Input Value
Char data typepublic class example { public static void main(String[] args) { char a='x'; char b='y'; System.out.println("char: "+a); System.out.println("char: "+b); } }
Let’s Run the code

Output Value

Short data type
The short data type is a 16-bit signed two’s complement integer.
Size: 2 byte or (16 bits)
Values: -32, 768 to 32, 767 (inclusive)
Default Value: 0
Syntax
short shortVar;
Example of Short data type
Input Value
Short data typepublic class shortvariable { public static void main(String[]args) { short a = 44; short b = 52; System.out.println("x:"+a); System.out.println("y:"+b); } }
Let’s Run the code
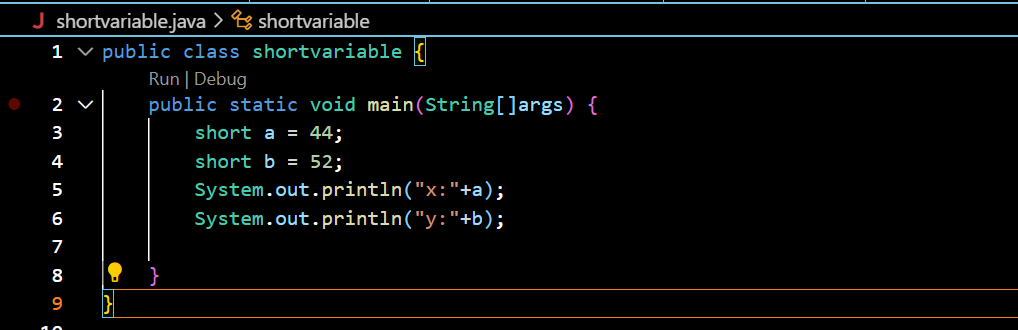
Output Value

Int data type
It is a 32-bit signed two’s complement integer.
Size: 4 byte or ( 32 bits )
Values: -2, 147, 483, 648 to 2, 147, 483, 647
Default Value: 0
Syntax
int intVar;
Example of int data type
Input Value
Int data typepublic class intvariable { public static void main(String[] args) { int a=10; int b= 12; System.out.println(" "+a); System.out.println(" "+b); System.out.println(" sum of two num : "+(a+b)); } }
Let’s Run the code

Output Value

Long data type
The range of a long is quite large. The long data type is a 64-bit two’s complement integer.
Size: 8 byte (64 bits)
Values: {-9, 223, 372, 036, 854, 775, 808} to {9, 223, 372, 036, 854, 775, 807}
Default Value: 0
Syntax
long longVar;
Example of long data type
Input Value
Long data typepublic class longvariable { public static void main(String...k) { long a=4500000; long b=-4466854; System.out.println("num1 : "+a); System.out.println("num2 : "+b); System.out.println("sum of two num : "+(a+b)); } }
Let’s Run the code

Output Value

Float data type
The float data type is a single-precision 32-bit IEEE 754 floating-point
Size: 4 byte (32 bits)
Values: upto 7 decimal digits
Default Value: 0.0f
Syntax
float floatVar;
Example of Float data type
Input Value
Float data typepublic class floatvariable { public static void main(String[] args) { float a=12.2f; float b=8f; System.out.println("num1: "+a); System.out.println("num2: "+b); System.out.println("sum of two num : "+(a+b)); } }
Let’s Run the code

Output Value

Double data type
The double data type is a double-precision 64-bit IEEE 754 floating-point.
Size: 8 bytes or 64 bits
Values: Upto 16 decimal digits
Default Value: 0.0d
Syntax
double doubleVar;
Example of double data type
Input Value
Double data typepublic class doublevariable { public static void main(String[] args) { double a=454847.15645; double b=42.25488559; System.out.println("num : "+a); System.out.println("num : "+b); System.out.println("sum of two num : "+(a+b)); } }
Let’s Run the code
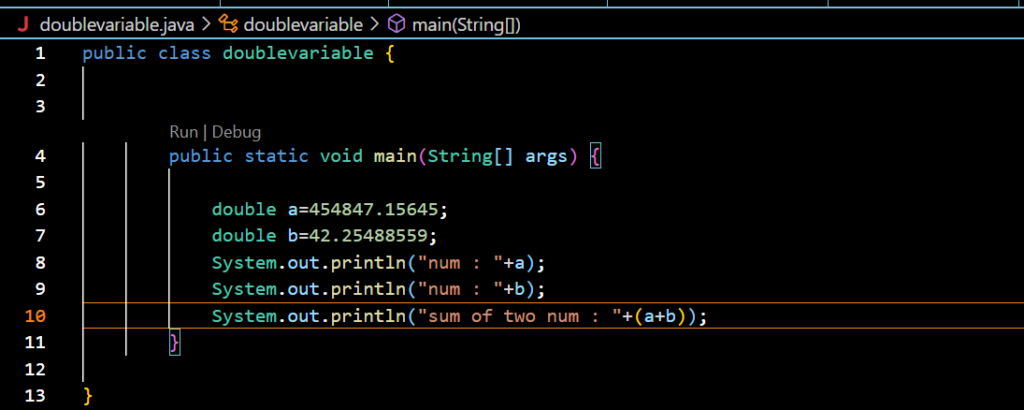
Output Value

If you have any queries regarding this article or if I have missed something on this topic, please feel free to add in the comment down below for the audience. See you guys in another article.
To know more about JAVA Wikipedia please click here .
Stay Connected Stay Safe, Thank you
0 Comments